In this article, we are going to write a simple C program, to calculate the sum of all the digits in the given number. For example, if we have the number 1234, then the sum of all the digits in the given number comes out to be 10. We will have a simple approach to achieving our goal here. We will understand the logic of the program, and then we will write a C program for the same. In order to understand the program completely, one needs to understand the concepts like –
Sum of Digits Program in C
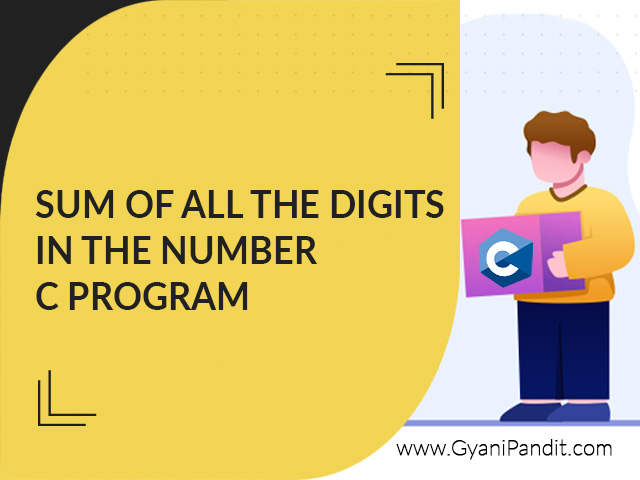
- while loop
- operators.
Sample Input output examples –
Example 1 –
Input:
Enter a number: 1234
Output:
10
Example 2 –
Input:
Enter a number: 8278
Output:
25
How to calculate the sum of all the digits of a number?
Now, When we have understood what we are required to do, let’s have a look at how can we do it, or what are the steps to get to the output of the program.
- First of all, we are going to get the number as user input.
- We would have a variable mysum, which would be initialized to 0, and it is going to hold the sum of all the digits from the given number at the end of the program.
- We have to get one digit at a time, and then we have to add it to the value of mysum. So, this seems to be a repeated task, and for that, we are going to make use of a while loop here.
- So, while our number does not become zero, we are going to do a few things –
- first of all, we would get the last digit, using the modulus operator.
- After that, we would add that last digit to the value of mysum.
- When we are done with that digit, we would simply get rid of that digit, by dividing the number by 10.
- Once the number becomes zero, we are out of the loop. Once we are out of the loop, we have our sum of all the digits in the number, stored in the variable mysum.
- So, we would just print the value of mysum as our output of the program.
If the above set of steps seems to be confusing, do not worry, since many things can get clear through the program. So now, let’s have a look at a simple C program to calculate the sum of all the digits of a given number.
C program to calculate the sum of all the digits in the given number –
#include<stdio.h>
int main()
{
int number, mysum = 0;
// we need to get the user input…
printf(“Please enter the number:”);
scanf(“%d”, &number);
/* while the number is not zero
we take the last digit of the current number
and add it to value of mysum
and then get rid of that digit by dividing the number by 10 */
while (number!=0)
{
int remainder = number%10;
mysum +=remainder;
number/=10;
}
// the sum of all the digits is now in mysum
printf(“The sum of all the digits is: %d”, mysum);
return 0;
}
As you can see in the above program, we are taking the number as user input, and then we are looping till the number is not zero, and in the loop, we are just taking the last digit, adding it to the value of mysum. After we are done adding the digit, we are getting rid of it, by dividing the number by 10.
Let’s have a look at the output of the above program now –
Output 1 –
Please enter the number:1234
The sum of all the digits is: 10
Output 2 –
Please enter the number:18278
The sum of all the digits is: 26
As you can see from the output, we had to enter the number, and then we get the sum of all the digits of the number. You can also try the program for some other values.
Conclusion
In this article, we have understood and implemented a simple C program, to calculate the sum of all the digits in the given number. For this, we made use of the while loop. You can also try to implement the program yourself, and you can try many other similar programs.
FAQ About Sum of Digits Program in C
Q: How to get the last digit of some given number?
Ans: To get the last digit of some number, we just get the remainder while dividing the number by 10.
Q: how to get the first digit of some given number?
Ans: To get the first digit of some given number, we just need to divide the number by 10, till the number becomes less than 10. Once the number becomes less than 10, we have only one digit, and that digit is our first digit.