In this article, we are going to understand the map function in Python. The map function is very important and useful at times. We would go through some examples and cases where you might want to use the map function over anything.
Map Function in Python
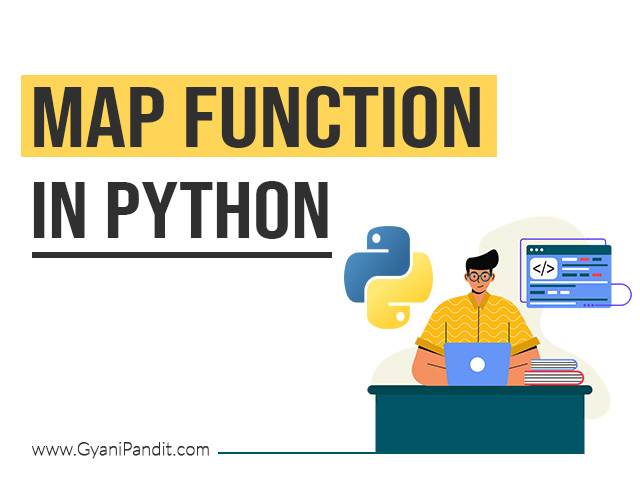
What is a map function in Python?
If you have heard about the map function for the first time, you might be wondering what is the map function. Well, the map function is a built-in function, which applies the given function on all the items of the given iterable.
Once we would have a look at different examples, you would get the importance of the map function and might want to use it in different situations.
How to use the map function?
Now, that we have got brief information about what the map function is, now we need to see how can we use the map function, so as to get familiar with the function and implement it as per our requirement.
Sometimes, we are in a situation, where we need to perform the same operations, on all the items from an iterable, to build a new iterable. One easy solution here is to make use of them for a loop. But we can also do this without using an explicit loop, just by using the built–in map function.
Let’s have a look at a situation, where we are trying to do the same operations on all the items in the iterable, and then we would try to make use of the map function to do the same thing.
In the below program, we are having a list with some numbers, and then we want to get another list, with the squares of all the numbers. We can do this easily using the for a loop. Here is the program for the same.
numbers = [1, 2, 3, 4, 5, 6]
square_nums = []
for number in numbers:
square_nums.append(number*number)
print(square_nums)
As you can see in the above program, this is the approach that we followed, to get a new list, with the square values for the given list items.
- We have a list with some numbers.
- We create another list, in which, we are supposed to store the squares for all the items.
- After that, using the for loop, we are appending the square value of each item in the new list.
- Once we are out of the loop, we can just print the new list as our output.
Let’s have a look at the output of the above program –
Output –
[1, 4, 9, 16, 25, 36]
As you can see, we got the new list, with the square values of the items in the given list. So, this was pretty easy using the for a loop. But we can do this without using the for loop as well. For that, we just need to make use of the map function.
Let’s have a look at a simple program, in which, we make use of the map function for the same task that we did above. Through this program, you would also get to know about the syntax of the map function, and what are the arguments that we provide to the map function.
def square(number):
return number * number
numbers = [1, 2, 3, 4, 5, 6]
square_nums = list(map(square, numbers))
print(square_nums)
As you can see, in the above program, we did the same thing, but this time, we used the map function. First of all, let’s have a look at the output of the above program, and then we would discuss what is going on here.
Output –
[1, 4, 9, 16, 25, 36]
As you can see, we have no difference in the output. So now, it is time for us, to understand what is going on here.
So, first of all, we have a list, with some numbers. The thing is that if you observe the map function that we are using in the program, you can find that we are passing two arguments there, first is the function object, and another is the iterable(list in our case). Note that we are not calling the square function there, but we are just passing the function object.
This is like, the square function that is going to be applied to each item in the iterable. In other words, the square of each number in the list would be calculated.
The map function returns a map object, and we are converting it into a list so that we can have a list.
So, from this program, we hope that you have understood the use case of the map function, and the syntax of the map function.
Using the map function with built-in function in python
In the previous example, where we understood the map function and its syntax, we used the user-defined function there. But here, in this example, we are going to make use of a built–in function. In this example, let’s say that we have a list of some integers, and then we need to have a new list, with the binary equivalent of each item in the given list.
For that, we have a built–in a function called bin(), which we are going to use here.
When we pass an integer to the bin function, it returns a string as the binary representation of the given integer.
So, let’s have a look at the below program, in which, we use the map function with the built-in function.
integers = [10, 20, 30, 1, 2, 3]
binary_numbers = list(map(bin, integers))
print(binary_numbers)
As you can see in the above program, we have the list with a few numbers, and then we are using the map function, to which, we are passing the bin function object(again note that we are not calling that function, just passing the function object. )
With the map function, we get a map object, and then we are converting it to a list. Let’s have a look at the output now.
Output –
[‘0b1010’, ‘0b10100’, ‘0b11110’, ‘0b1’, ‘0b10’, ‘0b11’]
As you can see, we got a list of strings as the binary representation of the given integers. So, in this way, we can use the built–in function with the map function.
Map Function Example
In the previous examples, we have seen the syntax and quite understood the working of the map function, but let’s have a look at another example, through which, we can understand the map function in a better way, and get familiar with it.
In the below program, we are going to have a list of strings, and we need to convert each string into uppercase. For that, we are going to create a function in our program. Let’s have a look at the below program.
def ConvertToUpper(s):
return s.upper()
names = [“GyaniPandit”, “Python”, “Programming”]
capital_names = list(map(ConvertToUpper, names))
print(capital_names)
As you can see in the above program, we have a list, with some strings. Also, we are having a function, which helps us convert our string to uppercase. So, we are using the map function, so that we can apply the ConvertToUpper function to each member in the list.
Let’s have a look at the output now –
Output –
[‘GYANIPANDIT’, ‘PYTHON’, ‘PROGRAMMING’]
As you can see in the list, we have got each string in the list converted into uppercase. So, we have successfully performed a simple program, in which, we have used the map function, to get a list with the strings converted to uppercase.
Using Python Map Lambda function
We know that we need to pass a function object to the map function. So now, we are going to learn to use the lambda function(anonymous function) with the map function. In the following example, we are going to use the lambda function inside the map function. The program is easy, in which, we are going to have a list with some numbers, and then we need to have another list, with the squares of each number in the given list.
Let’s have a look at the simple program now –
numbers = [1, 2, 3, 4, 5, 6]
square_nums = list(map(lambda x:x*x, numbers))
print(square_nums)
As you can see in the above program, we have made use of the lambda function, to get the square of the given number, and otherwise, all the other things are the same. Let’s have a look at the output of the above program –
Output –
[1, 4, 9, 16, 25, 36]
As you can see, we got the desired output. So, we can also use the lambda function with the map function.
Note that when we are using the map function, we need to pass a function object, and iterable. The iterable can be a list, tuple, etc.
Conclusion
We have seen much about the built–in map function in Python. The map function applies the given function to all the items in the iterable. You can make use of the map function as and when required. To the map function, we need to pass a function object and an iterable.
FAQ About map function in Python
Q: What is a map function in Python?
Ans: Using the map function, we can execute the specified function for each item in the iterable(list, tuple, etc.).
Q: What does the map function return?
Ans: The map function returns a map object(an iterator), after applying the given function to each element in the given iterable.