In this article, we are going to understand and perform a Python program, to calculate the factorial of some number, using recursion. In order to understand the program in a better way, one needs to be familiar with the concepts like –
- What is factorial?
- What is recursion?
So, you can explore these concepts if you are not already familiar with them, but here, since we are going to write a program here, we would understand these terms in brief.
Factorial of a Number using Recursion in Python
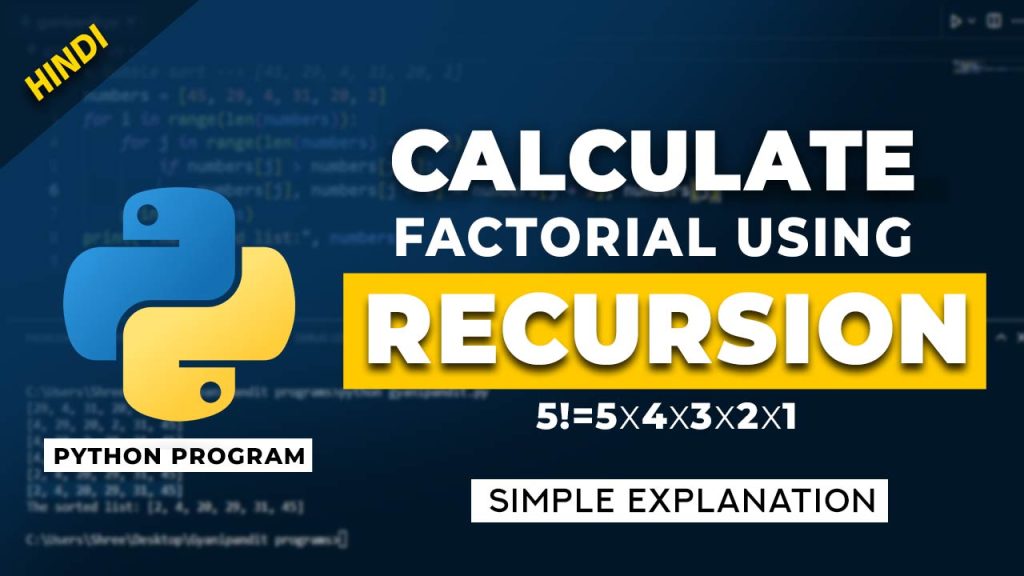
Sample Input Output –
Example 1 –Ā
input:Ā
Enter a number: 5
Output:Ā
Factorial = 120
Example 2 –Ā
input:Ā
Enter a number: 10
Output:Ā
Factorial = 3628800
What is factorial?
We can understand the term factorial as the product of that number, and all the integers below that number. For example, if we want to calculate the factorial of 5, it comes out to be 5x4x3x2x1, which is 120. So, in this way, you can calculate the factorial of some given number.
What is recursion?
We are required to calculate the factorial of some number, and that too, using recursion. Recursion is a very important and useful concept when it comes to programming. In simple terms here, we can understand recursion as ā a function calling itself. So, we are going to write a function here, in which, we are going to again call the same function.
Once you get to visualize the program here, you would understand recursion here.
So now, that we have very briefly understood what is factorial and recursion, let’s have a look at how are we going to achieve our goal.
Steps to calculate the factorial of some number using recursion –
- We are going to get the number as user input, whose factorial is to be calculated.
- After that, we have a function, which would help us calculate the factorial of that number, so we need to call that function, passing that number.
- This function is going to return us the factorial of that number.
Let’s have a look at the function that is going to help us to calculate the factorial of the number –
def factorial(number):
if number == 1 or number == 0:
return 1
else:
return number * factorial(number-1)
As you can see in the above function, we are passing a number to the function, and if the number we are passing is 0 or 1, then we are returning 1 as the factorial value, since the factorial of 1 is 1, and for 0 as well, the factorial is 1. So, this becomes the base condition, or the situation, where we need to stop.
Let’s say that we need to find the factorial of 5. In such a situation, the expression with the if evaluates to False, and we move to the else block, where we are again calling the function, to calculate the factorial of 4. This makes sense because if we have a careful look at the formula to calculate the factorial, then we can find that the factorial of n is equal to n multiplied by the factorial of (n-1). So, this is what we are doing. The base condition is there so that we can stop the recursion.
So now, let’s have a look at a simple python program, through which, we are going to calculate the factorial of a given number. We have already seen the function, and we are just adding some lines for taking user input and showing the output.
def factorial(number):
if number == 1 or number == 0:
return 1
else:
return number * factorial(number-1)
num = int(input(“Please enter the number to calculate the factorial:”))
fact = factorial(num)
print(f”The factorial of {num} is {fact}”)
As you can see in the above program, we are having the function to calculate the factorial of some number. After that, we are just getting the number as user input, after which, we are calling that function, and it returns the factorial of the number. After that, we are just showing the factorial of the number as output.
Let’s have a look at the output of the above program –
Output –
Please enter the number to calculate the factorial:5
The factorial of 5 is 120
As you can see, we asked for the factorial of 5, and we got the factorial of 5 as 120. You can try executing the above program for different outputs. You can also try checking for some conditions, where the user enters some negative number. Have a look at the below program, in which, we are giving some messages if a negative number comes as input from the user.
def factorial(number):
if number == 1 or number == 0:
return 1
else:
return number * factorial(number-1)
num = int(input(“Please enter the number to calculate the factorial:”))
if num>=0:
fact = factorial(num)
print(f”The factorial of {num} is {fact}”)
else:
print(“Invalid input”)
As you can see in the above program, we have just made some changes, and now, if the user enters a negative number, then the message will be shown as – āInvalid inputā.
You can also try to execute the program for some different values, and observe the output.
Conclusion:
In this article, we have understood and implemented a simple python program, to calculate the factorial of some given number, using recursion. We understood recursion in brief here, but recursion is a very broad topic, and you can further explore recursion. You can also try other such programs related to recursion.
FAQ About Factorial of a Number using Recursion in Python
Q: How to calculate factorial without recursion in Python?
Ans: In python, we have a simple function called factorial, using which, we can simply calculate the factorial of some given number.
Q: What is recursion?
Ans: We can understand recursion as a technique, in which, we are creating a function, which calls itself, till it reaches the desired output.
Q: What is factorial?
Ans: The factorial of some number can be calculated as a product of that number, and all the integers below it. For example, the factorial of 4 is 4x3x2x1, which comes out to be 24.