In this article, we are going to understand f-strings, or formatted string literal, which is a great way to format strings. Other than this, we have the format method as well, but the f-string is more readable and more concise than the other ways for formatting, and also they are faster.
It is quite similar to what we do while we make use of the format method. We would consider using some examples, so that we can properly understand the concept, and can use it as and when required in our Python programs.
F-strings in Python
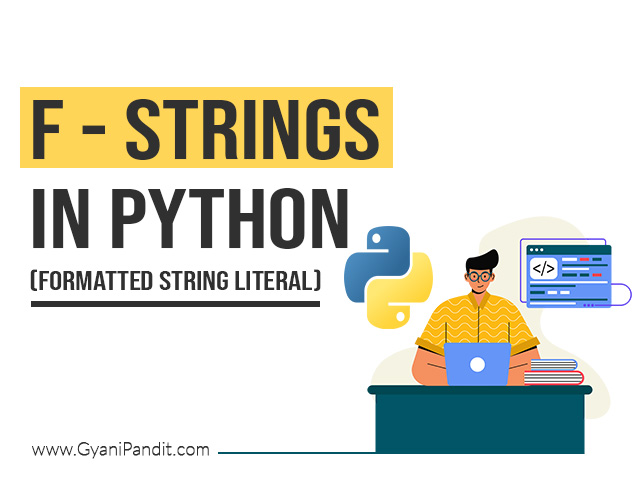
How to create F – string in Python programs?
As mentioned earlier, the f strings or formatted string literal is a great way to format strings. The thing is that if we were to use the format method to format the strings, then it would look something like this. Let’s have a look at a simple program, where we are using the format method, and then we will have a look at the f – string.
user_name = “GyaniPandit”
message = “Hello {}”.format(user_name)
print(message)
As you can see in the above program, we have a user name, and then we have a message, where we are using the format method. Here, we have a replacement field(placeholder), where, we are going to have the user_name, according to the format method that we are using here. After that, we are just printing the message.
Output –
Hello GyaniPandit
Now, let’s try the same thing with help of the f – string. But the question is that how can we create the f – string? Well, it is easy, and we just need to write f or F before the string, and we need to use the curly brackets as placeholders that contain the expression, which would be replaced with the value.
Let’s have a look at a simple program, through which, we can understand how can we use the f string, or formatted string literal.
user_name = “GyaniPandit”
message = f”Hello {user_name}”
print(message)
As you can see, we again have the user_name, and then we have a message, where we are trying to format the string, using the formatted string literal. As can be seen in the program, it is more readable, and more understandable.
Let’s have a look at the output of the above program –
Output –
Hello GyaniPandit
As you can see, we are getting the formatted string as our output. Let’s have a look at another example, through which, we can understand the concept in a better way.
user_name = “GyaniPandit”
user_age = 25
print(f”User name is {user_name} and age is {user_age}”)
As you can see in the above program, we are trying to format the string, using the f string. You can see that it is much more readable and understandable, and it is very easy when we have multiple expressions to be replaced. So, using the f strings is much more convenient in many situations.
Even we can also include any valid python expression here since the f strings are evaluated at runtime. So, this allows you to do something like this –
print(f”{20 * 5}”)
Including this simple instruction in the program, and executing then, we can find that we get the result of the expression.
Calling functions/methods with f-strings
Now, when we have been exploring the f-strings, we have only seen values of the variables or some simple expressions here.
But we can also call some functions/methods with the f strings. So, let’s have a look at a simple program, which, we are going to call a simple function.
list1 = [1, 2, 3, 4, 5, 6]
print(f’the length of the list : {len(list1)}’)
As you can see in the above program, we have a simple list, with 6 items in it. After that, we are trying to format a string, and in there, we are calling the len function, so as to get the length of the list. So, the f – string is much more convenient to use at times.
So, as per the requirement, you can call the user-defined functions here as well, and get the desired output.
Conclusion
In this quick tutorial, we understood a great way to format strings, which is f-strings, or formatted string literal in Python. You can explore the f-strings, and can make use of the f – string to format the string as per your requirement in the programs.
FAQ about F-strings in Python
Q: How to create an f string in Python?
Ans: To create an f string in python programs, we just need to prefix the string with the letter ‘f’ or ‘F’.
Q: What is the format method in python?
Ans: Using the format method, we can format our string, putting the specified values in the string’s placeholders. We use the format method for string formatting.