In this tutorial, we are going to learn about the if-else statements in C++. At times, in real life, we come across situations, when we need to do something, on the basis of some condition. For example, consider this statement – “If it is cold, wear a sweater”. So, here, wearing a sweater is an action, but it is only being done, if it is cold. So, just like this, there are many other situations, in which, we would be doing something, on the basis of some condition.
Similar to this, in programming as well, we have to deal with some situations, in which, we would do something, on the basis of some condition. So, for that, we can use the if else statement. So now, let’s explore the if-else statements in C++.
If else statements in CPP
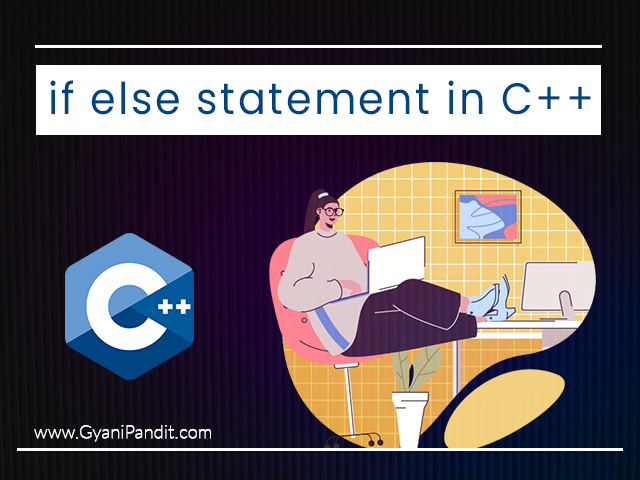
In programming, we come across situations, when we need to do something, on the basis of some condition. In such situations, we can make use of the if-else statements in C++. For example, if the user enters the correct login credentials, then only let him/ her login, or if the user age is 18 or more, and he/she has a voter ID, then only he/she can vote, or if the number is even, then do something.
So, let’s first have a look at the syntax of the if else statement, and then, we will have some examples, going through which, you would get familiar with the concept, and be comfortable using it in your C++ programs.
#include <iostream>int main()
{
if (condition)
{
// this block executes if condition is true.
}
else
{
// this block executes if condition is false.
} return 0;
}
As you can see from the above syntax, we have all the other basic things that we have in the boilerplate, and along with it, we have the if keyword, after which, we are just writing some conditions (you will get this from an example ahead), and then, we have a block(surrounded by curly brackets), within which, we would have some code. Now, this block executes, if the specified condition is true. Just below that, we have the else keyword. This else executes when the condition with the if becomes false.
This specifies that we are doing something like – “if the condition is true, do this, else do that”.
So, the “do this” code is in the if block, and the “do that” code is in the else block.
Note that it is not necessary to give the block after the if and else keywords unless you have more than one instruction executing with the block. Let’s have a look at a simple example, through which, we would understand how to use the if-else, and how to specify the condition.
In the below program, we are just checking if the number entered by the user is odd, or even. Just the condition is that if the remainder when we divide the number by 2 should come to 0, we can say that the number is even. Here’s the program –
#include <iostream>int main()
{
int number;
std::cout << “Please enter the number:” << std::endl;
std::cin >> number;
if (number%2 == 0)
std::cout << “The number is even!” << std::endl;
else
std::cout << “The number is odd!” << std::endl; return 0;
}
As you can see from the above program, we are just getting a number as user input, and then, we are simply checking if the number is even, through the condition. Now, if the condition is true, the instruction after the if statement would execute, otherwise, the instruction after the else keyword would execute.
Here is the output of the above program –
Please enter the number:
4
The number is even!
As you can see, for the entered number, it is able to tell whether the number is even or odd. So, this is how you can use the if-else statements in our programs.
Notice that we haven’t given the block after the if and else statements. This is because it is not necessary if you are going to execute only one instruction in the if, and in the else. But if you have more than one instruction executing on the basis of condition, then you should give the blocks. Here is an example of that.
#include <iostream>int main()
{
int number;
std::cout << “Please enter the number:” << std::endl;
std::cin >> number;
if (number%2 == 0)
{
std::cout << “The number is even!” << std::endl;
std::cout << “The number is divisible by 2” << std::endl;
}
else
{
std::cout << “The number is odd!” << std::endl;
std::cout << “The number is not divisible by 2” << std::endl;
} return 0;
}
As you can see from the above program, this time, we need a block after the if keyword, and the else keyword, because we have multiple instructions to execute.
Let’s have a look at the output of the above program –
Please enter the number:
11
The number is odd!
The number is not divisible by 2
As you can see from the output, since the number we entered was odd, the else block was executed. Since we had all the instructions in the block, they were executed. If you forget the block, then only the immediate instruction after the if statement would execute. So, be careful, and if you are having more than one instruction to execute if the condition is true, then do not forget to have a block.
Summary
In this tutorial, we have seen the if else statement in C++. At times, we can find these if-else statements useful, when we need to do something on the basis of some condition. We have seen some examples, through which, we can easily understand the concept, and use it later as and when required in our C++ programs.
If you find this tutorial to be interesting, you can explore our other tutorials related to C++ programming language to learn more about C++ programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to if else statements in C++
Q: What is an if-else statement in C++?
Ans: In C++, when we are in such a situation when we need to do something on the basis of some condition, at that time, we can make use of the if-else statements.
Q: Is if a keyword in C++?
Ans: Yes, if is a keyword in C++, which is used as a conditional statement, when we need to do something on the basis of some condition.
Q: Is it compulsory to write else after if?
Ans: Well, the corresponding else block executes if the condition in the if statement is false. So, it is ok if we are just writing if block, and not the else block. But the else block cannot be there without the if block.