In this tutorial, we are going to learn about the if-else ladder in C++. You might be already familiar with the if-else statements in C++. They are at times useful when we need to do something on the basis of some condition. This is like – “if this condition is true, do this, else do that.”. This is how we use the if-else things in our C++ programs.
But in this tutorial, we are going to move further and discuss the if-else ladder in C++. When you would see the example, you would understand why are we having this concept to life in C++.
if else ladder in CPP
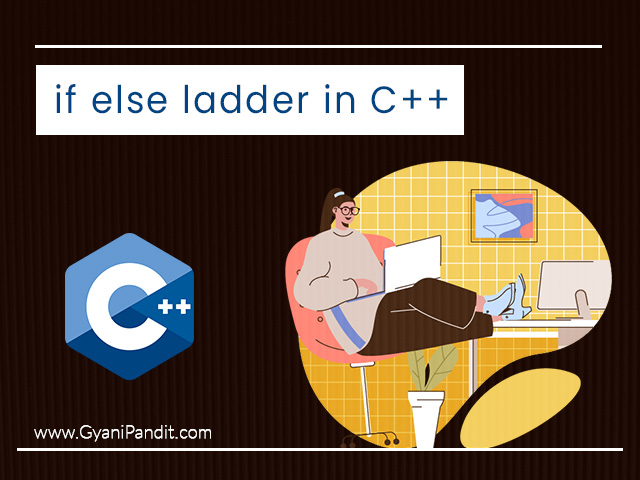
In situations, when we need to do something on the basis of some condition, we make use of the if else statement in C++. But if you have observed the if else statement in the C++ programs, you might have observed that with the if keyword, we are writing the condition, and when this condition is false, we are executing the block with the associated else keyword. So here, we are not checking the condition after the else keyword.
But at times, you might want to check the condition after the else keyword again. What should we do in such situations? Well, the thing is that we write the condition after the if keyword traditionally, so, if we write the if keyword after the else keyword, and then give the condition, we would be able to check another condition. So, this is the if-else ladder. Let’s have a look at the syntax for the if-else ladder –
#include <iostream>int main()
{
if (condition)
{
// code here executes if condition is true
}
else if (condition)
{
// Code here executes if condition is true
}
else
{
// code here executes if the condition from the above if is false.
} return 0;
}
As you can see from the syntax, it just looks like the normal if-else stuff, but the thing is that here, we are writing if after the else again, to check another condition. To demonstrate the need for this, let’s have a look at a simple example. In his example, let’s check if the input number is positive, negative, or zero.
#include <iostream>int main()
{
int number;
std::cout << “Please enter the number:n”;
std::cin >> number;
if (number > 0)
{
std::cout << “The number is positive.n”;
}
else if (number < 0)
{
std::cout << “The number is negative.n”;
}
else
{
std::cout << “The number is zero.n”;
}return 0;
}
As you can see from the above program, we have the if-else ladder. Here, first of all, we are getting the number as user input, and then we are first checking if the number is greater than zero. If this condition gets false, we move to the corresponding else, where again, we are checking another condition, that if the number is less than zero. Now, if this condition also gets false, we again have the corresponding else, which would be then executed.
Let’s have a look at the output now –
Please enter the number:
12
The number is positive.
As you can see from the output, we were first asked for a number as user input, and then, we got the output according to what number we had input. So, this is kind of an easy example. Let’s have a look at one more example, for becoming more familiar with the concept, and using it in your C++ programs.
In the following program, we are going to get the marks out of 100 as user input, and then according to the input, we are going to decide whether the student gets A, B, C, D, or F grades. Have a look at the program.
#include <iostream>int main()
{
int marks;
std::cout << “Enter your marks out of 100: “;
std::cin >> marks; if (marks > 100)
{
std::cout << “Invalid score entered!n”;
}
else if (marks >= 90)
{
std::cout << “You got A Grade!n”;
}
else if (marks >= 75)
{
std::cout << “You got B Graden”;
}
else if (marks >= 50)
{
std::cout << “You got C Graden”;
}
else if (marks >= 35)
{
std::cout << “You got D Graden”;
}
else
{
std::cout << “You got F Graden”;
} return 0;
}
As you can see in the above program, we are getting the marks out of 100 as user input, and then, we are giving the grade as output accordingly.
Let’s have a look at the output of the above program –
Enter your marks out of 100: 87
You got B Grade
Note that we have set up a simple grading system, and this is not so out of the box, but you can set up some different grading systems if you want. But it is essential to understand the if-else ladder concept so that we can use it as and when required.
Summary
In this tutorial, we have seen the if-else ladder in C++. The concept is very essential, and at times useful. It helps us when we need to again check conditions in the else. You can solve some more examples related to this concept so that you can become familiar with the concept, and be comfortable to use the concept ahead in your C++ programs.
If you find this tutorial to be interesting, you can explore our other tutorials related to C++ programming language to learn more about C++ programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to if else ladder in C++
Q: What is the if else statement in C++?
Ans: The if else statement in C++ is used often when we are required to do something on the basis of some condition.
Q: How do we check the condition after else?
Ans: Well, if we need to check some condition after the else, we just need to write the if keyword, followed by the condition. This is the if-else ladder.