In this tutorial, we are going to learn about nested if-else statements in C++. Well, you might already be familiar with the concept of if-else, and its need. At times, when we need to do something, on the basis of some condition, we make use of the if-else statements. Now, in many situations, we might also require to nest them, which essentially means that we might need to write some if block, inside some if block for example.
We will go through different examples, to understand the concept, and then you can use it as and when required in the C++ programs.
Nested if else statements in CPP
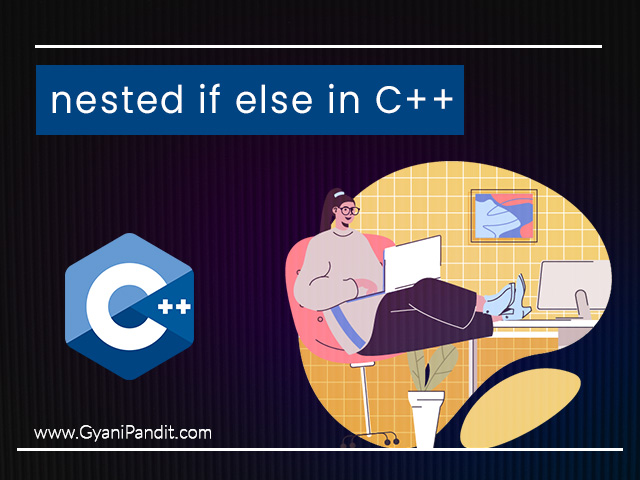
As mentioned earlier, nesting of if else essentially would mean that we might need to write an if block, inside another if block. But what is the need? Well, there are situations, when we might need to check some more conditions when we are executing the if block, or the else block. So certainly, in such situations, we would make use of the if statements again to check further conditions.
Let’s understand this thing with an example.
#include <iostream>int main()
{
int number;
std::cout <<“Please enter a number:”;
std::cin >> number;if (number!=0)
{
if (number>0)
{
std::cout <<“The number is positiven”;
}
else
{
std::cout << “The number is negativen”;
}
}
else
{
std::cout << “Number is zeron”;
}return 0;
}
Have a look at the above example. In this example, we are checking if the given number is positive, negative, or zero. For this, we have made use of the nested if statement. First of all, we are getting the number as user input, and then, we are first checking if the number is not zero. If this condition is true, then we are moving in the block, and again check if the number is greater than zero. If this is again true, we print that the number is positive.
So, you can try executing the above program, and this is the sample output for it –
Please enter a number:123
The number is positive
As you can see, we entered a positive number and got the output as the number is positive. You can try for other input numbers as well, and observe the result.
So, we hope that you have understood the need for the concept. So now, let’s also have a look at using logical operators, to check on multiple conditions.
For this, we will perform a simple program, in which, we are going to find the biggest number out of the three given numbers.
#include <iostream>
int main()
{
int num1, num2, num3;
std::cout << “Please enter the first number:”;
std::cin >> num1;
std::cout << “Please enter the second number:”;
std::cin >> num2;
std::cout << “Please enter the third number:”;
std::cin >> num3;
if (num1>num2 && num1>num3)
{
std::cout << num1 << ” is largest!”;
}
else if (num2>num3)
{
std::cout << num2 << ” is largest!”;
}
else{
std::cout << num3 << ” is largest!”;
}
return 0;
}
As you can see from the above program, we are just finding the largest of the given three numbers. So, some of the first lines just take the 3 numbers as user input. After that, in the first, if statement, we are writing the conditions, for checking if num1 is greater than num2, and num3. This is like if num1 is greater than both, num1 is the greatest.
So here, we are using the logical AND operator, for the multiple conditions. If both the conditions are true, then only that if block would execute.
On the other hand, if any of the conditions is false, then we move to the corresponding else block, and we are again checking the condition if num2 is greater than num3. So, this is how the program follows.
But it is essential to understand that we can make use of the logical operators here when we need to check with multiple conditions. You can solve some more examples, to get more familiar with the concept, and be comfortable using the concept ahead in the C++ programs.
Summary
In this tutorial, we learned about nested if statements in C++. At times, we might need to use the nested if statements in our C++ programs. You can simply try to solve more and more examples, to get familiar with the concept, and later on, you can use the concept in other C++ programs.
FAQs related to Nested if else in C++
Q: What is the if keyword in C++?
Ans: The if keyword in C++ is used when we need to do something on the basis of some condition.
Q: Can we write else without the if keyword?
Ans: else block is executed when the previous condition with if gets false. So, to write the else part, you have to have the if part. So, it is not possible to write else without an if.
Q: What is nested if else in C++?
Ans: Nested if else essentially means that we are writing an if block inside an if block. This is at times required in our C++ programs.