In this article, we are going to learn about how can we create an empty set in Python. Set is one of the built–in datatypes in Python, which can be used to store collection of data. The thing is that in the set, we cannot have duplicate elements. At times, we might need to create a new empty set in Python. We would have a look at examples, to demonstrate how can we create an empty set.
How to create an empty set in Python
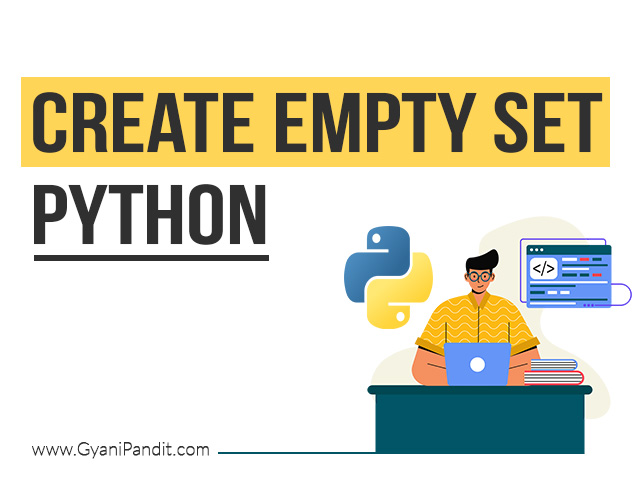
How to create a set?
To create a set in Python, we have multiple ways, like –
- Putting the set items into curly brackets
- Using the built–in set() function.
Have a look at the below program, in which, we are trying to create a set, using both the above-mentioned ways.
set1 = {1, 2, 3, 4, 5}
set2 = set([5, 4, 3, 2, 1])
print(type(set1))
print(type(set2))
As you can see in the above program, we have created two sets here, in which, one is created by putting items within curly brackets, and the other one is created using the built–in set function.
You can try executing the above program, and find that we have created the sets.
But now, we are more interested in creating an empty set, so now, let’s explore that.
How to create an empty set?
Usually, when we create a set, we make use of the curly brackets, within which, we have the elements. But this time, we need to create an empty set. So, if we are just using the curly brackets, and not putting any elements into it, then we end up creating an empty dictionary, and not a set. Let’s have a look at a simple example, through which, we can understand the same thing.
numbers = {1, 2, 3, 4, 5}
print(type(numbers))
As you can see in the above program, we have curly brackets with some elements inside them. In this situation, if you check the type of object here, you would see that you get a set, which is perfectly fine and usual.
But now, let’s say that we remove all the elements within the curly brackets, and then try to check the type of the program. We might expect an empty set, but what we create is an empty dictionary.
numbers = {}
print(type(numbers))
As you can see here, this time, if we try to execute the above program, we can see in the output, that we have created a dictionary. We are checking the type of the object using the type() function. So, now we know that using the empty curly brackets won’t help in creating an empty set. In order to create an empty set, we need to make use of the built–in set() function.
Have a look at the below program, in which, we have created an empty set, using the built–in set() function.
set1 = set()
print(type(set1))
As you can see, we are using the built–in set function, to create an empty set. You can execute the above program, and find that we are able to create an empty set using the built–in set() function. We are using the type function, to check the type of the object.
Conclusion
We have seen how to create a new empty set in Python. To create an empty set, we just need to use the built–in set function. You can try to execute the above programs to understand the same thing. Note that we can also create a set by putting the items within curly brackets. But, just using the empty curly brackets won’t help us create an empty set.