In this article, we are going to understand the __str__ method in Python. This method can be said as the dunder str method, where the dunder means double underscores. These methods with double underscores are also called magic methods.
So, the __str__ method returns the string representation of the object. Basically, the __str__ method is called when the functions like print, or str are invoked on the object. Other than that, we also have the __repr__ method, and if the __str__ method is not defined, then calling the print, or str function will call the __repr__ method. You can explore the __repr__ method more, but here, we are going to stick to understanding the __str__ method.
What is an str method in Python?
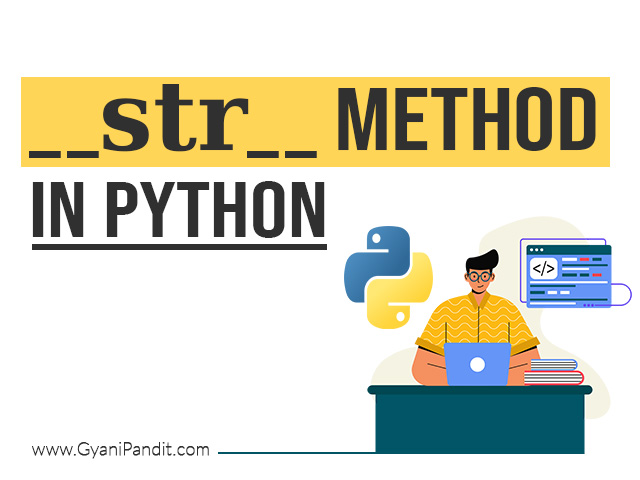
Default program example(without writing the __str__ method) –
Now, we are just going to dive into some practical stuff, through which, you can get the use of __str__ method. First of all, let’s have a look at a simple program, in which, we are not going to write the __str__ method, and we would try to print the object using the print function.
class Book:
def __init__(self, name, pages, price) -> None:
self.name = name
self.pages = pages
self.price = price
book1 = Book(“The book1”, 100, 56)
print(book1)
As you can see in the above program, we have a class Book, and then we also have the __init__ method. Then we are creating a Book object, and then we are trying to print it. If you try to execute the above program, you would see some weird output, which may not be what we want(It is the default representation of our object). If we are printing the Book object in the above example, we might want to have some information about the Book, and instead of that, we are getting some weird output(it is just the default representation of the objects).
But let’s say that we want some information about the object when we print the object. So, we know that when we call the print function, the __str__ method is called. So, in our Book class, we just need to write the __str__ method. So, let’s do it now.
Program using the __str__ method
class Book:
def __init__(self, name, pages, price) -> None:
self.name = name
self.pages = pages
self.price = price
def __str__(self) -> str:
return f”The name of book: {self.name}nNumber of pages:{self.pages}nPrice:{self.price}”
book1 = Book(“The book1”, 100, 56)
print(book1)
As you can see in the above program, we just have created the __str__ method. The __str__ method returns the string, which is a kind of informal string representation of some object. After that, we created a Book object, and we are printing the object. This time, this is the output that we get –
Output –
The name of the book: The book1
Number of pages:100
Price:56
As you can see, we got some information about the Book object this time, when we are printing the Book object.
Also, we have mentioned above, that when we are using the str function, then also the __str__ method gets called. So now, let’s have an example, which tries to demonstrate the same thing.
class Book:
def __init__(self, name, pages, price) -> None:
self.name = name
self.pages = pages
self.price = price
def __str__(self) -> str:
return f”The name of book: {self.name}nNumber of pages:{self.pages}nPrice:{self.price}”
book1 = Book(“The book1”, 100, 56)
info = str(book1)
print(info)
As you can see in the above program, we just have made a small change, where we have called the str function, and then we are just printing the information. In this situation as well, we get the same output as before. Have a look at the output –
Output –
The name of the book: The book1
Number of pages:100
Price:56
As you can see, we have got the same output this time as well. So, the __str__ method returns the string representation of the given object. This is a kind of information about the given object.
Some brief about __repr__ method
Now, that we have understood much about the __str__ method, let’s discuss in brief the __repr__ method. As mentioned earlier, the __str__ method is invoked when the print/str functions are used. If we have not written the __str__ method, then the __repr__ method is called. So, let’s define the repr method alone now, and let’s see what is the output.
class Book:
def __init__(self, name, pages, price) -> None:
self.name = name
self.pages = pages
self.price = price
def __repr__(self) -> str:
return f”Book({self.name}, {self.pages}, {self.price})”
book1 = Book(“The book1”, 100, 56)
print(repr(book1))
print(str(book1))
print(book1)
As you can see, this time, we have written the __repr__ method. Basically, according to the documentation, the __repr__ is used for the official string representation of an object. The thing is that if we have defined the __repr__(), but not __str__(), then the __repr__() is also used when some informal string representation of the object is required.
Let’s have a look at the output of the above program.
Output –
Book(The book1, 100, 56)
Book(The book1, 100, 56)
Book(The book1, 100, 56)
As you can see, calling the repr(), str(), or print, results in the same output. So, this is the __repr__ method, which is used for the official string representation of an object.
Conclusion
We have understood the __str__ method, which is a dunder method in Python. It is useful when we need to have an informal string representation of our object. We can use the __str__ method with our classes and return some useful information related to the object whenever needed. We have seen some examples, to understand the __str__ method, and also we have seen the __repr__ method in brief. You can explore more about the __repr__ method.
FAQ About str method in Python
Q: What is the str method?
Ans: The str method is used to return the string representation of the object.
Q: When is the str method called?
Ans: The str method is called when the print() or str() function is invoked on an object.
Q: what is the repr method in Python?
Ans: the repr method is used for the official string representation of an object. It should be a valid python expression, which can be used to create an object again.