In this article, we are going to learn about raw strings in Python. Raw strings in Python are created by prefixing the string literal with ‘r’ or ‘R’. The useful thing about the raw string in Python is that the raw strings treat backslash as a literal character. This is useful at times, when we are required to have the backslash as the backslash, and not the escape character in the string.
We would have a look at some of the examples, so that we can understand the raw strings in Python, and then we can implement it in our program, as and when required.
Raw strings in Python
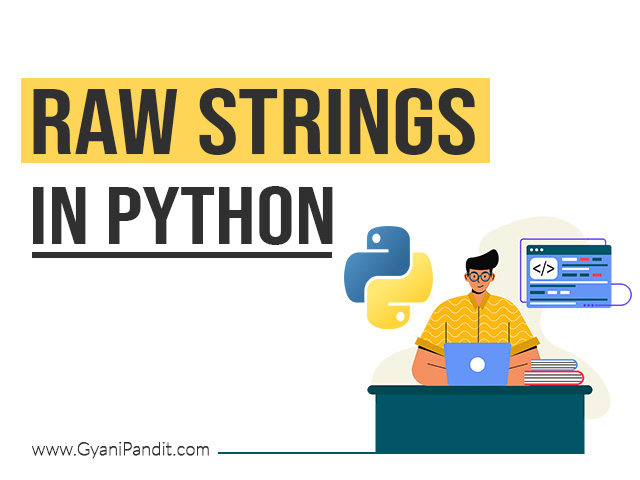
How to create a raw string?
To create a raw string, we just need to prefix the string literal with ‘r’ or ‘R’. Also, if we are having a backslash in the string, and we are creating a raw string, then the backslash will be treated as a backslash, and not an escape character. In the below program, we have a normal string, in which, we are using the backslash, lets have a look at the program and the output, so that we can get an idea.
my_string = “Hello from GyaniPanditnwe are on the next line.”
print(my_string)
As you can see, in the above program, we have a string, and there we are using the n, which simply means a newline, when we are using a normal string. If you have a look at the output of the above program, you can find that the things after the n are on the new line. Lets have a look at the output –
Output –
Hello from GyaniPandit
we are on the next line.
But now, lets consider that we are taking a raw string instead of the normal one. So, the backslash here will be considered as backslash, and not an escape sequence. So, here is the program for the same.
my_string = r”Hello from GyaniPanditnwe are on the next line.”
print(my_string)
As you can see in the above program, instead of a normal string, we have made use of the raw string. Remember that to create a raw string, we need to prefix the string with r or R. Also, in the string, we are using the n here, but here, the backslash is a literal character, so we would not see the newline now, but instead, we would see the n as it is in the string.
Lets have a look at the output now –
Output –
Hello from GyaniPanditnwe are on the next line.
As you can see in the output, we have the n as it is, since we are using the raw string this time. Lets have a look at another program, through which, we are trying to understand the use the raw string.
my_string = “Hello from GyaniPanditttwe are learning about raw string!”
print(my_string)
As you can see in the above program, we have a normal string, and we are trying to use a t, which means a tab, in the string. So, if you try to have a look at the output of the above program, it comes out to be something like this –
Output –
Hello from GyaniPandit we are learning about raw string!
As you can see, we could see two tab spaces here, since the string is normal. But if the string was raw string, the backslash would be considered as a literal character, and we won’t see the tab spaces.
Have a look at the below program, which demonstrates the same.
my_string = r”Hello from GyaniPanditttwe are learning about raw string!”
print(my_string)
In the above program, we have made use of a raw string instead of a normal string. So, lets have a look at the output now –
Output –
Hello from GyaniPanditttwe are learning about raw string!
As you can see, since we are using the raw string, the backslash is considered as a literal character.
Importance of using raw string
Well, as of now, you might have got the concept about the raw strings. In here, raw strings are created by prefixing the string by r or R. In the raw strings, the backslash is treated as a literal character. But now the question is, when are the raw strings useful?
Well, in the situations when we need to deal with the backslashes in the string, we can make use of the raw strings. For example, lets consider the file/directory path in Windows. Lets have a look at the below program, in which, we are having a path of a file.
file_path = r”C:UsersGyaniPanditDesktopPythonPrograms”
print(file_path)
As you can see, in the above program, we have the file path, in which, we have the backslashes in the string. But here, we would want the backslash to be considered as literal character, and in such a situation, we can make use of the raw string.
You can execute the above program, and have a look at the output, and you can find that we could have the file path. But if you try to make the above string as normal string, and then try to execute it, it would run into error.
Conclusion
We have explored about the raw string in Python. The raw string can be created by prefixing the string with ‘r’ or ‘R’. We can create the raw strings in our python programs, as and when required. At times, it is useful for us, to create raw strings in our python programs. We have gone through different examples, demonstrating the use and the need of raw strings in Python.
FAQ About Raw strings in Python
Q. How to create raw strings in Python?
Ans: To create a raw string in Python, we just need to prefix the string with ‘r’ or ‘R’.
Q: Why do we use the raw string?
Ans: We use the raw strings, as in the raw strings, the backslash is considered as a literal character, and there are situations, in which, we might want that the backslash should be considered as a literal character.
Q: When do we use raw strings in Python?
Ans: We can use the raw strings in Python, in the situations, when we need to deal with strings, containing backslashes, and we want the backslash to be considered as literal character.