At times, in our python programs, especially in the case of mutable objects, we need to have some copy of objects, so that we can make changes to the copy, without affecting the original one. But when it comes to copy, you might have heard that we can have a shallow copy or a deep copy. For example, when you use the copy method for the list, you get a shallow copy of the list.
But what do we mean by deep copy? Well, in this article, we are going to understand deep copy. We would go through some examples, so as to get a clear picture of the deep copy.
What is a deep copy Python?
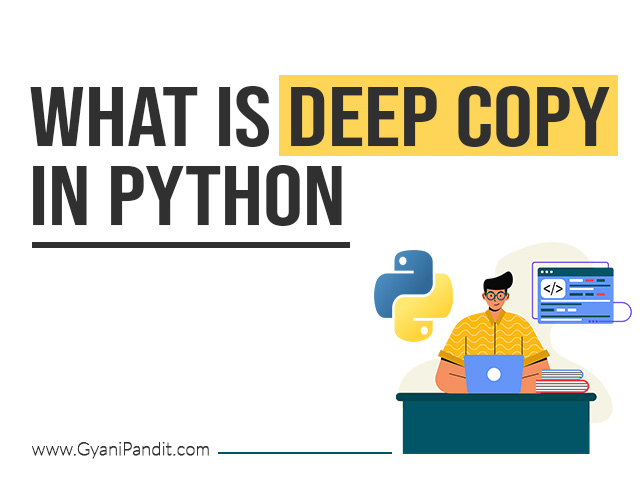
What happens when you use = in Python?
You might have used the assignment operator in Python a lot, but the thing is that if you want to create a copy, doing a simple assignment is not going to do what is required. For example, if we have this list –
list1 = [1, 2, 3, 4, 5]
And then we try to create a new reference variable, and assign the list1 to that variable –
list2 = list1
With the above operation, someone might mistakenly think that we have got a copy here. But the thing is that now, both the reference variables are pointing to the same list. So, any changes made through one variable would reflect through another variable as well, because both are pointing to the same list.
So, with this, we are clear that just doing the simple assignment is not doing what is required.
What is deep copy?
Let’s stick with the above example of lists. Let’s say that now you want to create a copy of the list, so you have the copy method with you. But the thing is that the copy method would give you a shallow copy of the list. By shallow copy, we simply mean that we have a new object, which contains references to the objects found in the original.
If it confuses you, you can just think that in the case of a list, we are having another list, with references to the objects found in the original list.
But here, when we create a deep copy, we get a new object, which has copies of the objects that are found in the original object. So, if we are creating a deep copy of a list for example, then we have another list, which contains copies of the objects that are found in the original list. The new object is created, and the copies of the objects found in the original are inserted recursively.
How to create a deep copy?
Now, that we have a brief idea about what is a deep copy, let’s have a look at how to create a deep copy. To create a deep copy, we can make use of the copy module. We just need to use the deep copy function. Let’s have a look at the below program, in which, we are going to create a deep copy of a list.
import copy
list1 = [1, 2, 3, 4, [5, 6, 7]]
list2 = copy.deepcopy(list1)
print(list2)
As you can see, we have a list(actually there is a list inside the list, so this is a mutable object, containing another mutable object). After that, we are creating a deep copy, using the deep copy function. After that, we are printing the list2 here. If you try to execute the program, you can see that we have got a copy of the list.
But then, the question arises what is the difference between shallow copy(the one which we get from the copy method), and deep copy(that we are getting here)?
What is the difference between shallow copy and deep copy?
The basic difference between shallow copy and deep copy lies in how we define shallow copy and deep copy. Let’s have a look at both –
- In shallow copy, we get an object, with references to the objects found in the original object.
- In a deep copy, we get an object, with copies of objects that are found in the original. The new object is created, and the copies of the objects found in the original are inserted recursively.
As you can see, in the case of shallow copy, we get another object, which has references to the objects found in the original object.
For example, if we had created a shallow copy for the above list, which contains another list, and tried to make changes to the nested list, have a look at what happens.
list1 = [1, 2, 3, 4, [5, 6, 7]]
list2 = list1.copy()
list2[-1][0] = 100
print(list2)
print(list1)
In the above program, we have a list, which contains another list. After that, we are creating a shallow copy of the list, using copy method. Then, we are trying to make changes to the first element of the list which is inside, and that too for the copy. So, we expect that the original should not be affected since we have created a copy. But after that, we are printing both the original list and the copy as well.
If you have a look at the output, you can see that we made changes to the copy, but the original list also got affected. This happened because when we created a shallow copy here, we got a new object, with references to the objects that are found in the original.
On the other hand, let’s try to perform the deep copy operation on the same list. Let’s make some simple changes to our program –
import copy
list1 = [1, 2, 3, 4, [5, 6, 7]]
list2 = copy.deepcopy(list1)
list2[-1][0] = 100
print(list2)
print(list1)
As you can see, in the above program, now we are creating a deep copy. Again we are then trying to do the same things. But this time, if you have a look at the output of the program, you would see that the original list was not affected this time, because when we created the deep copy, it creates a new object, and then recursively inserts copies of the objects found in the original object.
Have a look at the output –
[1, 2, 3, 4, [100, 6, 7]]
[1, 2, 3, 4, [5, 6, 7]]
As you can see, the copy list was not affected this time, since we created a deep copy. So, as and when required, you can create a deep copy.
Conclusion
We hope that you have understood what is deep copy in Python, and also the basic difference between shallow copy and deep copy. As and when required, you can create shallow copies or deep copies of the objects.
FAQ About deep copy python
Q: What is a deep copy in Python?
Ans: In a deep copy, a new object is created, and then recursively copies of the objects found in the original object are inserted.
Q: What is a shallow copy in Python?
Ans: In the shallow copy, a new object is created, and then the references to the objects found in the original object are inserted.
Q: What is the difference between shallow copy and deep copy in Python?
Ans: In shallow copy, we get a new object, into which, the references of objects found in the original object are inserted. On the other hand, when it comes to deep copy, a new object is created, and recursively, the copies of the objects found in the original object are inserted.