In this article, we are going to understand how can we get a random number in Python. At times, we are in situations, when we need to have a random number in our Python programs. In such situations, we have the random module to our rescue. So here, we are going to understand how to get a random number in Python. We would go through some different examples, so as to get a clear picture of the concept.
How to get a random number in Python?
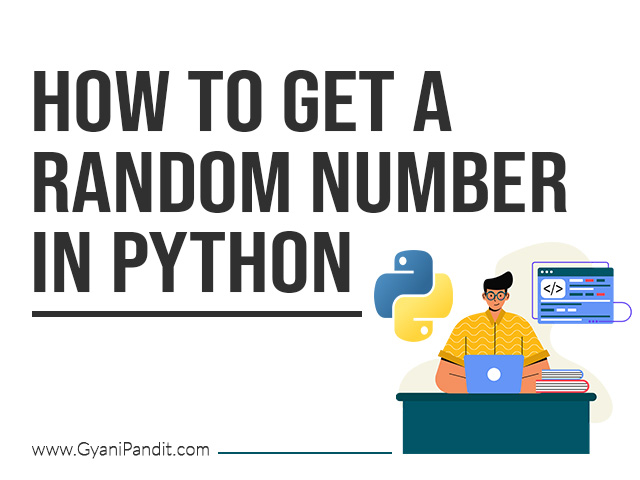
To get a random number in Python, we can just make use of the random module. There are several things that we can do, to get a random number. In this article, we are going to mainly focus on getting a random floating point number in the range [0.0, 1.0). Also, we would have a look at how can we get a random integer in the given range.
Here are the functions that we are going to use –
- random() → this function returns a random floating point number in the range [0.0, 1.0).
- randint(a, b) → this function returns a random integer N, such that a <= N <= b.
So now, let’s have a look at how to get random numbers using the above-stated functions.
Getting random floating point number in the range [0.0, 1.0)
We can make use of the random() function to get a random floating point number in the range [0.0, 1.0).
So, whenever you need some random floating point number, you can make use of the random() function. The random function returns a floating point number in the range [0.0, 1.0), but you can modify the value as required. Let’s have a look at a simple program, which tries to demonstrate the same thing –
import random
print(random.random())
As you can see in the above program, we just have two lines. First of all, we need to import the random module, so we did that, and in the next line, we are just using the random() function, to get the random number in the range [0.0, 1.0). You can try executing the program for some time and observe the random numbers as outputs in the stated range.
Also, you can modify the random number that you get from the function, as per your requirement.
Getting a random number in the given range
Now, if you want to get the random integer in some given range, we can simply make use of the randint function here. To the randint function, we need to give the values a and b, and the function returns a random integer in the given range.
Now, let’s have a look at a simple program, in which, we would try to get a random integer in the given range.
import random
number = random.randint(1, 10)
print(number)
As you can see in the above program, we are trying to get the random integer in the range of 1 to 10. You can try to execute the above program, and observe the output for random numbers in the range.
Conclusion
So, in this way, we can get random numbers. As and when required, you can use the functions from the random module, and get the random number as required. We have seen the random() function to get the random floating point number in the range [0.0, 1.0). On the other hand, we have seen the radiant (a, b) function, using which, we can get the random number in the given range.
FAQ About How to get a random number in Python
Q: How to get a random integer in python?
Ans: We can make use of the randint() function from the random module, to get the random number in the given range.
Q: How to get a random element from a sequence?
Ans: To get a random element from a non-empty sequence, we can just make use of the choice function from the random module, which returns a random element from the non-empty sequence.
Q: What is pseudorandom number?
Ans: We can understand the pseudorandom number as the number which is computed by certain algorithms.