In python, if we want to store a collection of data, we can make use of the list. But the thing is that, in the list, we can have data of multiple data types. So, within a list, we can have integers, we can have strings, Booleans, another list, etc. But if you are familiar with some other programming language, like Java or C, you might have seen that there is something called as an array, in which, we can store data of similar datatype.
But when it comes to listing, as mentioned earlier, we can store data of multiple datatypes as well. So, in python, if we are required to create an array(having an array functionality), we are required to do some things, and this is what we are going to explore here. We are going to have a look at what are arrays in Python, and how can we create an array in Python.
How to create an array in Python
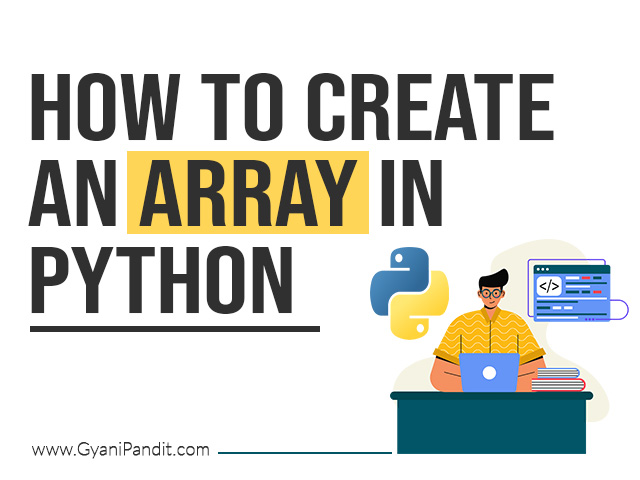
What is an array in Python?
Well, an array in general is a collection of items that are stored at contiguous memory locations. The thing is that in the array, we can store data of similar datatype. To create an array in python, we can make use of the array module, or we can even use NumPy to create an array(ndarray). We would go through both ways to create an array and will see some examples, to have a clear understanding.
Creating an array using the array module –
In Python, we can create arrays using the array module, so we just need to import the array module. You can use different instructions to import the array module you can import the entire module or just the necessary stuff from the module.
Here is the quick syntax of how can we create an array –
import array
variable = array.array(typecode, value_list)
Here, with the typecode, we are specifying the type of objects that we want here, and then, we are providing the initializer(values).
We are going to have a look at a simple program, to create an array in Python. But before that, we need to be familiar with the different typecodes that we can use here. The typecodes are just used to specify the types of objects stored in the array. Here is the table for the same. You can find the table in the documentation as well.
Typecode | C Type | Python Type | Minimum size(bytes) |
‘b’ | signed char | int | 1 |
‘B’ | unsigned char | int | 1 |
‘u’ | wchar_t | Unicode character | 2 |
‘h’ | signed short | int | 2 |
‘H’ | unsigned short | int | 2 |
‘i’ | signed int | int | 2 |
‘I’ | unsigned int | int | 2 |
‘l’ | signed long | int | 4 |
‘L’ | unsigned long | int | 4 |
‘q’ | signed long long | int | 8 |
‘Q’ | unsigned long long | int | 8 |
‘f’ | float | float | 4 |
‘d’ | double | float | 8 |
So now, let’s have a look at a python program to create an array –
from array import *
arr = array(‘i’, [1, 2, 3, 4, 5])
print(arr)
As you can see in the above program, we have imported everything from the array module, and then we have a simple instruction to create an array. We are using the array constructor, in which, we are first giving the type code (in our case, the type code is ‘i’, which means signed integer). The other argument is the values that are stored in the array in square brackets(the initializer basically).
You can try executing the above program and observing the output. Now, let’s have a look at another program, in which, we would try to store something else in the array, other than the allowed type.
from array import *
arr = array(‘i’, [1, 2, “3”, 4, 5])
print(arr)
As you can see in the above program, we are saying that the array is going to contain integers, and we are giving the values, in which we have a string as well. So in such a situation, we get errors. You can try executing the program and observing the error.
So, you can try creating an array, using the array module. Whenever you are required to have an array functionality, you can just choose to create an array using the array module. You can explore the array module in detail, but here, we have just learned how can we create an array using the array module.
Creating an array using NumPy
Using NumPy, we can create an array, and now, we are going to have a look at how can we create an array using the NumPy library. The NumPy Library is very vast, so you can explore it for other things, but here, we are going to have a look at how to create an array using the NumPy library.
Using NumPy, we can get some additional functionalities. So, we would at times, want to use the NumPy library for creating arrays. The thing is that if you already have python, you can install NumPy by using the following instruction –
pip install numpy
or you can also use Anaconda, which is very easy to get started. Now, when we have the NumPy installed, we can just import it into our program, and use the things. So now, let’s try to create an array using NumPy.
First of all, we just need to import NumPy, and then, we would create an array, using the array function. Let’s have a look at the below program, through which, we can understand creating an array using NumPy.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr)
As you can see, we have imported NumPy as np(np is the alias for NumPy). After that, we are using the array function to create an array. To create an array, we are passing it a list here, and it would be converted to an array.
So, this way, using NumPy as well, we have created an array. So, as and when required, you can make use of the NumPy library to create an array. NumPy is a very vast, and very important package, so you can explore it in detail, but here, we have learned to create an array using NumPy. Note that if you are completely new to NumPy, you might not have it installed already. So, you would first need to install it, in order to use it.
Conclusion –
In python, we have a list, if we want to have a collection of elements. But the problem is that in the list, we can store multiple types of data. But sometimes, we might want to have the array functionality, so, we can create an array using the array module as we have seen above. We can also make use of NumPy, to create an array.
FAQ About How to create an array in Python
Q: What is a list in Python?
Ans: In python, a list is one of the built–in datatypes, used to store the collection of data. We can store data of multiple data types in the list.
Q: How to create an array in Python?
Ans: We usually make use of the list in python, when we need to work with something array–like, but still, if we want to have the array functionality in python, we can make use of the array module, to create an array.
Q: What are the different ways to create an array in python?
Ans: When we need to store collection of data, we would think of an array generally. In python, we can make use of the list straightway, but if we want the array functionality in python, we can make use of the array module, to create an array. Also, we can make use of NumPy, to create an array.