In many different python programs, you might have seen the statement if __name__ == “__main__”. So here in this article, we are going to understand a special variable __name__. We would see some different examples, through which, we can understand what is __name__ variable, and this would also give us some idea about the instruction if __name__ == “__main__”, which we use in many times.
What is name variable in Python?
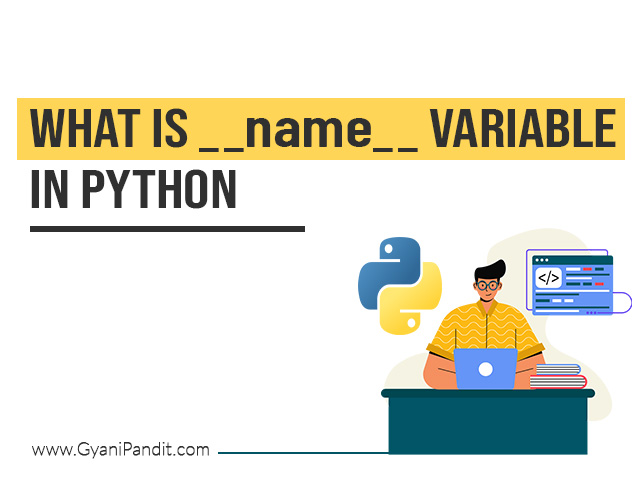
The __name__ variable in Python is a special variable. The value for the __name__ variable depends on how are we executing the containing script. At times, it is useful to make use of the __name__ variable, as you might have seen sometimes, in the statement if __name__ == “__main__”.
The question now is that what are the values contained by the special variable __name__. So now, let’s have a look at different conditions, in which, we can have different values for the special variable.
What values can the variable __name__ have?
The thing is that when the script is executed directly, the __name__ variable is set to ‘__main__’. On the other hand, if the file is imported as the module, then the __name__ variable is set to the name of the module. To understand this more clearly, let’s have a look at an example, which gives us a clearer idea about the scenarios.
Case 1 – When our python file is executed directly.
As mentioned earlier, when the python file is executed directly, the __name__ variable is set to ‘__main__’. So, let’s have a look at a simple example, which tries to demonstrate the same thing.
def dosomething():
print(“The value in the __name__ variable is: ” + __name__)
dosomething()
As you can see, in the above program, we just have a simple function, with the name do something, in which, we are printing the value of the __name__ variable. After that, we are just calling the do something function.
Let’s say that the name of this program is gyanipandit.py, and we are executing this program directly, then the __name__ variable is set to ‘__main__’. You can try to execute the program. So now, let’s have a look at the output from the above program.
Output –
The value in the __name__ variable is: __main__
As you can see, since we are executing the file directly, and not importing it, the __name__ variable is set to ‘__main__’.
Case 2 – When we import our python file into another file –
In the prior case, we saw that if we are executing the file directly, then the __name__ variable is set to ‘__main__’. But in this case, let’s consider that we are not executing the file directly, but importing it into another python file. In such a case, the __name__ variable is set to the name of the module. Let’s have a look at a simple program, through which, we can understand the same thing –
Let’s say that we have a program, with the name program.py. Here is the code for program.py –
def dosomething():
print(“The value in the __name__ variable is: ” + __name__)
if __name__ == ‘__main__’:
dosomething()
On the other hand, let’s say that we have another file gyanipandit.py, in which, we are importing our program file. Here is the code for the same.
import program
print(“We have imported the python file into another python file”)
program.dosomething()
As you can see in the above program, we have imported the program file. Then we are calling that do something function, and if we try to execute the file gyanipandit.py, you can find the output as something like this –
Output –
We have imported the python file into another python file
The value in the __name__ variable is: program
This last line of output comes from the program module. Since we are calling the do something method, in which, we are printing the value for the __name__ variable, and now, we find that since the program file is not executed directly, but imported into another python file, the __name__ variable was set to the module name.
Also, you can observe that in the code for the program.py file, we have used the statement if __name__ == ‘__main__’. With the help of this statement, we are simply saying that if this file is directly being executed, then only do the other stuff. This helps us when we want to import the file into another file, since on importing, we might not want to directly execute the things from the imported module.
Conclusion –
Here, we have seen the special variable __name__, We now know that the variable has a value ‘__main__’, if we are executing the file directly, and if the file is being imported, the __name__ variable is set to the module name. The __name__ variable is very useful at times.
FAQ About name variable in Python
Q: What is a name variable in Python?
Ans: The name variable is a special variable, which is set to ‘main‘ if the file is executed directly. On the other hand, if the file is imported, then the name variable is set to the module name.
Q: what is if name == ‘main‘ in Python?
Ans: In our python programs, we often use the if name == ‘main‘ statement. By doing this, we simply mean that do the further things only if the file is directly executed, and not imported.
Q: What is the use of name variables in python?
Ans: The name variable is a special variable that is set to ‘main‘, if the file is directly executed. But if we are importing the file, then the name variable is set to the module name.