In this article, we are going to understand and implement a very simple Java program, in which, we are going to Swap First and Last Digits of a Number in Java. We would, first of all, have a look at the steps to get to the output, and then we would have a look at the Java program for the same. In order to understand the program completely, one needs to have a good understanding of the following concepts –
- while looping in Java
- Math class in Java
- Operators in Java
So, have a look at the below input outputs, so as to have a clearer idea about what are we required to do.
Swap First and Last Digits of a Number in Java
Example input outputs –
Example 1 –
Input: 1234
Output: 4231
Example 2 –
Input: 27267
Output: 77262
So now, let’s have a look at what approach are we going to have, to solve this problem.
How to swap the first and last digits in the given number?
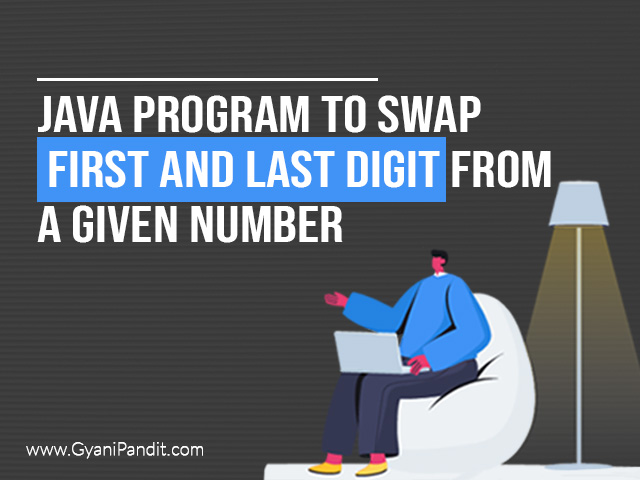
Now, that we have an idea of what are we going to do, let’s have a look at how are we going to do it. We just need to get the first digit, and the last digit, and swap them. The approach is very simple, and once you get the approach, you will be easily able to solve the problem by yourselves. Here is the approach –
- Get the number as user input. (You can initialize the value in the program as well)
- Getting the last number is easy! Just get the remainder while you divide the number by 10. (use the modulo operator)
- To get the first digit, we can have multiple approaches –
- Approach 1 – Keep dividing the number by 10, till the number becomes less than 10. Once the number is less than 10, we are only left with one number, which is the first digit.
- Approach 2 – Other than that, we can also make use of the Mathematical functions, to get the first digit. (this approach will be used in the program)
- After that, when we have got the first and the last digit, we need to remove them from our number. So for that, we are going to perform some operations, like firstly removing the first number, and then the last number(you can have a look at the code, through which you can simply understand what are we actually doing)
- After that, we just need to do some arithmetic operations, to get the number, with the first and last digits swapped.
- When we have the number with the first and last digits swapped, we just need to show it as the output of our program.
Let’s have a look at the program, in which we are trying to do the same –
Java program for swapping the first and last digits of the given number –
import java.util.Scanner;
public class Main
{
public static void main(String[] args) {
int lastDigit = 0, firstDigit = 0;
System.out.println(“Please enter the number:”);
Scanner scanner = new Scanner(System.in);
// we are getting the user input.
int number = scanner.nextInt();
// getting the last digit from the number.
lastDigit = number%10;
int power = (int) Math.log10(number);
// Getting the first digit from the number.
firstDigit = (int) (number / Math.pow(10, power));
int a = firstDigit * (int) Math.pow(10, power);
int b = number %a;
number = b/10;
number = (lastDigit * (int)Math.pow(10, power)) + number* 10 + firstDigit;
System.out.println(“The result: ” + number);
}
}
As you can see, in the above program, we are first taking the number as user input. Then, we are just getting the first and the last digits. After that, we are just doing some basic operations, to get to the output. Let’s have a look at the output of the above program –
Output –
Please enter the number:
1234
The result: 4231
As you can see from the output, we could easily swap the first and the last digit in the given number. The result is the number after swapping the first and last digits from the number. You can also try executing the above program, and observe the output.
Conclusion
In the above article, we have seen how to swap the first and last digits of a number. You can also try executing the program, and also observe the output. You can also try your hands on some other programs.
FAQ About Swap the First and Last Digits of a Number in Java
Q: How to get the last digit from the given number?
Ans: To get the last digit from the given number, we just need to get the remainder, when we are dividing the number by 10. For that, we can just make use of the modulo operator(%).
Q: How to get the first digit from the given number?
Ans: To get the first digit from the given number, there can be multiple approaches. One simple approach says that just keep dividing the number by 10, till the number becomes less than 10. Once the number is less than 10, we are just left with one digit, which is our first digit.