In this article, we are going to understand and perform a simple and interesting python program, in which, we would have a number, and we need to calculate the sum of all the digits in the given number. Have a look at the below examples first, which give an idea about what are we required to do.
Examples –
Number: 1234
Output: 10 (since the sum of all the digits in the number comes out to be 10)
Number: 1901
Output: 11
Number: 18924
Output: 24
As you can see in the above examples, we have the number, and as an output, we need to have the sum of all the digits in the given number. Let’s have a look at how can we achieve this task. We are going to write a python program now. Let’s first have a look at the complete program, and then we would try to understand what things are happening here.
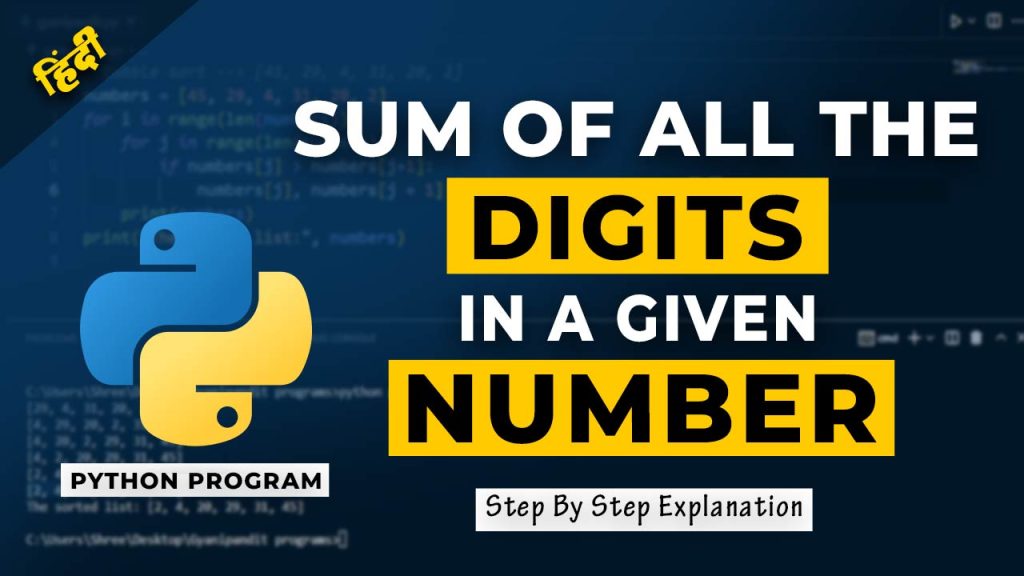
Sum of all the digits in a given number Python program
number = int(input(“Please enter the number: “))
mysum = 0 # this variable holds the sum of all the digits in the end.
temp = number
while temp!=0:
rem = temp%10 # get the last digit
mysum+=rem # add it to the sum
temp//=10 # get rid of the last digit since we are done with it.
print(f”The sum of digits in {number} is {mysum}”)
As you can see in the above program, we are taking a number as user input, and then we are trying to get the sum of all the digits in the given number. Let’s have a look at the output of the above program now –
Output Example 1 –
Please enter the number: 1234
The sum of digits in 1234 is 10
Output Example 2 –
Please enter the number: 18924
The sum of digits in 18924 is 24
As you can see, when we are giving some number, we are getting the sum of all the digits in the given number. Now, let’s try to discuss what is happening here.
Have a look at the below steps, which demonstrate how things are happening in the above program.
- First of all, we are getting the number as user input.
- We are creating a variable mysum, to hold the sum of all the digits from the given number in the end.
- As a next step, we are going to make another variable, to which, we are assigning the same data as the given number so that we can manipulate the copy of the data.
- After that, we loop till the number is not zero, and within that, we are doing some things repeatedly. Let’s have a look at them –
- Here, we are getting the last digit of the number, by getting the remainder when we divide that number by 10.
- When we have the last digit, we just add it to the sum.
- When we have added the digit to the sum, we are done with that digit and would want to get rid of that digit, which is why we are dividing the number by zero, so that we do not have that last digit in the next iteration.
- When the number becomes zero, we are out of the while loop.
- After that, we have the sum stored in the mysum variable, and we are showing the same thing as the output.
From the above simple steps, we can easily understand the program. Here, we just have understood and implemented a simple Python program, to calculate the sum of all the digits in a given number.
Conclusion
As you can see, this program is very simple to understand and implement. You can try implementing the program and try to have a look at the outputs, for different input values. Furthermore, you can try some other similar programs.
FAQ About the Sum of all the digits in a given number Python program
Q: How to get the last digit of some numbers?
Ans: You can get the last digit of some number, by getting the remainder when you divide the number by 10.
Q: How to calculate the sum of all the digits in the given number?
Ans: If you want to calculate the sum of all the digits in the given number, you can simply repeatedly get the last digit of the number, then add it to the sum, and then get rid of the last digit(since we have added that), till the number becomes zero. Once the number is zero, we have the sum of all the digits in the given number.
Q: How to get the first digit of some number?
Ans: If you want to get the first digit of some given number, you just need to divide that number repeatedly, till the number becomes less than 10. Once the number is less than 10, we only have one digit, which is our first digit.