JSON.Stringify ():
We are now familiar with the thing, that we use JSON for data transfer from/to a web server. The thing is that when we are sending data to the web server, the data needs to be a string. So, if you want to send some JavaScript object to the server, you would need to first convert it into a string, and this is what stringify method does for us.
Using the stringify method, we can convert the JavaScript object into a string. If you are still confused, do not worry, since we are going to look at an example, with which, many things would get clearer.
So, we are using the stringify method to convert our JavaScript object to a string. The stringify method also works on arrays. Now, let’s have a look at the example, through which, we can understand the stringify method.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta http-equiv=”X-UA-Compatible” content=”IE=edge”>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>JSON stringify</title>
</head>
<body>
<script>
var userData = {
userName:”GyaniPandit”,
followers:1000000,
posts:15000,
isVerified:true
}
var userDataString = JSON.stringify(userData);
console.log(userDataString);
</script>
</body>
</html>
As you can see, the above is a very simple program, where we have some simple HTML code, and within the script, we are having JavaScript. First of all, we have a simple JavaScript object, which has some key-value pairs. After that, we are using the JSON.stringify method, and pass the userData object as an argument. In this case, this object would be converted to a string, which follows JSON notation. After that, we are getting the string into the console. Let’s have a look at the output.
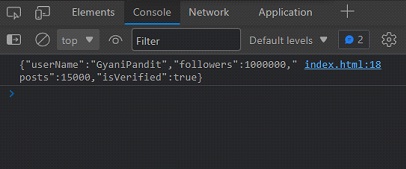
As you can see, we have the JavaScript object converted to the JSON string. As you can see, this string follows the JSON notation.
So, to summarize, the JSON.stringify method helps us to convert the JavaScript object to a string. So, whenever we are required to do so, we will make use of the JSON.stringify method.