JSON.parse():
The thing is that when we receive some data from the web server, it is always a string. When we parse the data with JSON.parse, the data becomes a JavaScript object. So, whenever we have received some string from the web server, and we need to make it a JavaScript object, we would simply use JSON.parse method.
Note: The written string is supposed to be in the JSON format, else we get a syntax error.
Let’s have a look at an example, through which, we can understand the JSON.parse method.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta http-equiv=”X-UA-Compatible” content=”IE=edge”>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>JSON parse</title>
</head>
<body>
<script>
mystring = `{
“userName”:”GyaniPandit”,
“followers”:1000000,
“posts”:15000,
“isVerified”:true
}`
myobject = JSON.parse(mystring);
console.log(myobject);
console.log(typeof myobject)
</script>
</body>
</html>
As you can see, in the above code, we have some javascript written. First of all, we have the JSON string, and then we are parsing the string with JSON.parse, and it becomes the JavaScript object, which means that we can do different things with the object. So, let’s have a look at the output now –
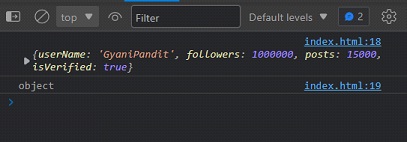
As you can see in the output, we have the object, and just below that, we have the type as the object as well. So, to summarize, when we receive some data from the web server, the data is as a string, and so, in our JavaScript program, we need to get it as an object. So, we make use of the JSON.parse method, and the data becomes a JavaScript object.