In this tutorial, we are going to learn about the syntax of a C++ program. Basically, you can consider syntax as some set of rules, which we need to follow or write, while we are writing our C++ programs.
We will understand this with help of a simple and basic C++ program, which would be eventually our first C++ program too.
CPP Syntax
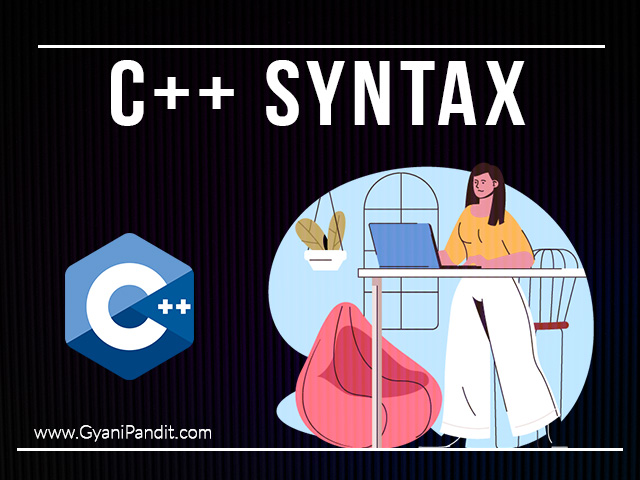
Let’s first have a look at the syntax of C++, and then we will try to understand the different things that we have in our programs.
#include <iostream>
using namespace std;
int main()
{
// some code here…
return 0;
}
As you can see in the above sample code, we have the syntax. So, let’s understand the different things that we have got here. These are some of the things, that you would regularly use in your C++ programs.
Including header files
Through the instruction #include<iostream>, we can include iostream header file, which contains the declarations of many functions and objects, which we can use in the program.
using namespace std → Using this line, we are just stating that make all the things from the std namespace available in this scope, without having to prefix std:: before them. Actually, using this is considered generally a bad practice, so we won’t be using this statement much often in the bigger programs.
main() function → the main function is the starting point of every C++ program. That is the main function that has to be there in every code. Our code will be there in the curly braces after the main function.
After that, we just have a single-line comment, which in this case, is there to tell us that there comes some code. It is basically a comment and is ignored by the compiler.
return 0 → this tells that the function has ended and the 0 here means success.
When you would go through many different programs in C++, you would become more familiar with the concepts and would be comfortable writing programs in C++.
When we will learn more concepts, you will get more and more familiar with the syntax and with further practice, things won’t feel like something weird.
So, eventually, the syntax of a C++ program would consist of all the things which are necessary for many programs from basic to advance.
Now, it is time to perform our very first program in C++. In this program, we are simply going to print a simple message on the console. If you are familiar with the C language earlier, you would know that we have printf function, but in C++, we can do this with the Cout. Let’s try this now.
#include <iostream>
int main()
{
std::cout<<“Hello from GyaniPandit”;
return 0;
}
As you can see in the above program, we have all the things from the syntax, (except the using namespace std instruction) and we are using the Cout, for giving some output to the console.
If you execute the above program, you can simply find that we are able to output some messages to the console.
Summary
In this tutorial, we learned about the syntax of our C++ program. It is important to understand the different things that are going to be encountered many times in your C++ programs, so that we can be comfortable using them, and not just write them just because we need to write them.
We also did our very first C++ program in this tutorial series, in which, we are simply trying to print some messages onto the console.
If you find this tutorial to be interesting, you can explore our other tutorials related to C++ programming language to learn more about C++ programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to C++ syntax
Q: What is iostream in C++?
Ans: It is a header file for dealing with a standard input-output stream. It contains declarations about some functions and objects used in the programs.
Q: What is Cout in C++?
Ans: Cout is an object, used along with the insertion operator(<<) in order to output a stream of characters.