In this tutorial, we are going to learn about Comments in C++. We will go through some simple examples, to understand the use and the need for comments.
Comments in cpp
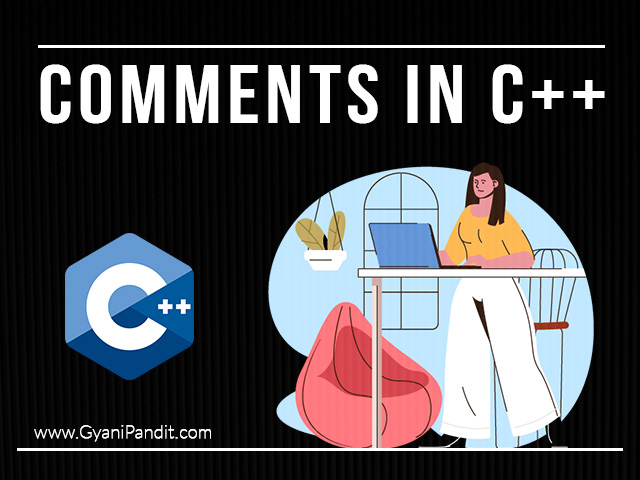
What are the comments in C++?
The comments in the C++ program are the lines that are written to explain something, some description about some block of code, some particular line of code, or the entire program.
The comments are ignored by the compiler. We often use the comments to explain what the block of code is or what the particular line is doing.
Also, there can be another use for comments. You might have read above, that the comments are ignored by the compiler, which means that even if we comment on some line or block of code, it would be ignored just like any other comment. So, when we want to prevent some code from execution, but want it in our program, we can simply comment on that code.
We will see some examples soon, to demonstrate these things.
Types of Comments in C++
There are two types of comments –
1. Single-line comments. –
These are the single-line comments, that is, comments in one line. These are denoted by “//” . You can find an example below, which demonstrates the single-line comments.
2. Multi-line comments –
Multi-line comments are comments that can be written in more than one line. So, anything that you write between the asterisk marks would be considered as a comment(/**/) for example, → /* this is a comment*/
Example for single-line comments in cpp-
#include <iostream>
int main()
{
int age = 30; // this variable holds age
std::cout<<“You are “<<age<<” years old!”;
return 0;
}
As you can see, the single-line comment is given with the //(forward double slashes). It is used to describe the line of code. This is a single-line comment, which means that in the same line, you write anything after the two forward slashes, and it would be considered a comment.
Example of multiline comment in cpp
#include <iostream>
int main()
{
/*
This is a multiline comment.
This is why it spans in multiple lines.
So, you can write some detailed description here.
*/
/*
In the below program, we just have an age variable,
and we are printing the age.
*/
int age = 30;
std::cout<<“You are “<<age<<” years old!”;
return 0;
}
As you can see, we have a multiline comment, which simply means that it is a comment, which spans multiple lines. So, when it comes to writing detailed descriptions about something, you can simply go with multiline comments.
For example for commenting on some code
#include <iostream>
int main()
{
int age = 30;
// std::cout<<“You are “<<age<<” years old!”;
return 0;
}
As you can see, in the above program, we have the age variable, with some number assigned to it, and then, we have an instruction for some output, but since it is commented, it would be ignored just like any other comment, and we won’t see any kind of output.
So, at times, when we want to keep some code in our program, but not execute it, we can simply comment on that code.
Why use comments in cpp?
Well, this is an obvious question, why should you use comments? There can be several reasons.
Interesting thing is that the comments are ignored by the compiler, but they are of great use to the one who is reading the code. they help in understanding the code. You can often use the comments so that if you give your code to someone, he/ she won’t annoy you by asking everything about how the code runs. As he/she would be able to read and understand the code.
In short, writing comments improves the readability of your code. It is not necessary to give comments for everything in your code, but it can be considered a good practice, to write comments in your code.
FAQs related to comments in C++
Q: What are the comments in C++?
Ans: Comments are used for writing some description about some block of code, line of code, or the entire program. They are simply ignored by the compiler.
Q: State types of comments in C++?
Ans: There are two types of comments in C++, single-line comments, and multiline comments. Single-line comments, as their name says, are comments which can be written on one line. On the other hand, if you need some comments that can span multiple lines, you can go with multiline comments.
Q: How to give comments in C++?
Ans: To give some single-line comments, you can use //(two forward slashes). Anything that you write after these two forward slashes would be a comment on the same line.
On the other hand, to write multiline comments, you have to include the comment between /* and */.