Constants in C
In this tutorial, we are going to learn about the constants in C programming language. At times, in our C programs, we might need to create constants. So, with examples, we will consider what are constants in C.
Constants in C
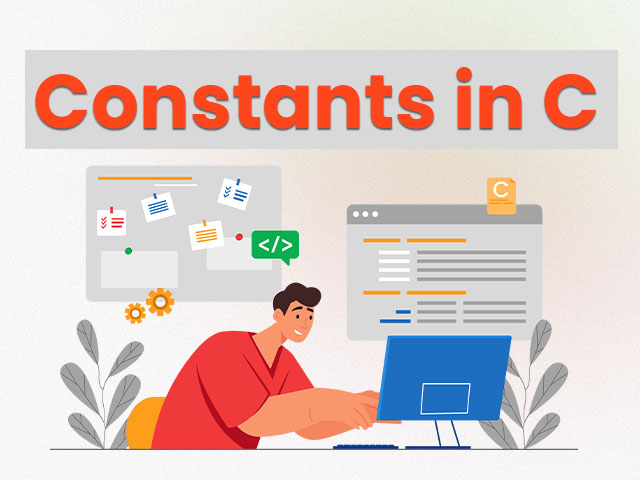
What are the Constants in C?
At times, when we need to store some data in our C programs, we make use of some variables. But if we are creating variables as we normally do in our programs, we can make changes to their values. But at times, we might be in some situation, where we might need to store some value in our program, and we also want it not to be changed throughout the program. In such a situation, we can make constants in our Programs.
We can consider constants as something, that cannot be changed throughout the execution of the program. It is at times required.
Creating some constant is very easy, and just using the const keyword, you can do it. Let’s have a look at a simple example, to understand how can we create some constants in our C program. Let’s say that we are writing a C program to calculate the area of the circle. We know that we have a constant value PI, which we would not want to be changed throughout the program. So, have a look at the program.
#include <stdio.h>
int main()
{
const float pi = 3.14159; // this value is approximate.
float radius = 3.5;
float area = pi * radius * radius;
printf(“Area: %f”, area);
return 0;
}
As you can see in the above program, we have created a constant, using the const keyword here. Other than that, we are just creating the pi variable as we normally do, except that we have added the const keyword before it, just to make it constant.
After that, it is a usual story, that we have another variable for radius, and then we are calculating the area, and storing it in the variable with the name area.
So now, since we have declared the variable as constant, the data won’t be modified throughout the program. Let’s have a look at another example, where we are creating a constant, and then we are trying to modify its value(which is basically not possible, and we are going to end up in error), but still, let’s try this!
#include <stdio.h>
int main()
{
const int number = 30;
number++;
printf(“Value of Number: %d”, number);
return 0;
}
As you can see in the above program, we are creating a constant, and then we are trying to increment the value of the constant, which is not possible, and we end up in error for doing this. You can try executing the program, and observe the error. This way, you can simply create constants in your C program, just by creating the variable as you usually do and adding the const keyword before it.
Creating constants using #define preprocessor directive
Other than using the const keyword to create constants, you can also make use of the #define preprocessor. Let’s have a look at a simple program, which demonstrates the same thing, and then we would see if should we use the #define preprocessor for creating constants.
#include<stdio.h>
#define Number 10
int main()
{
printf(“Value of Number:%d”, Number);
return 0;
}
As you can see in the above program, we have used the #define preprocessor directive. These constants have a global scope. This means that they can be accessed and modified from anywhere in the program. Also, there is no type checking, when you are creating constants, using the #define preprocessor directive. There can be some more drawbacks, but due to these drawbacks, you should consider thinking before you are using the #define preprocessor directive to create constants.
Summary
In this tutorial, we have learned about how can we create constants in our C programs. At times, it becomes important for us, to create constants in our C programs, and in such situations, we can make use of the const keyword, to create the constant, and you can also make use of the #define preprocessor directive.
Later on, when you would perform some more programs, and try creating some constants, you would get familiar with the concept, and be comfortable while using the concept next time. If you find this tutorial to be interesting, you can explore our other tutorials related to C programming language to learn more about C programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to constants in C
Q: What are the -constants in C?
Ans: We can say that the constants cannot be changed throughout the program. At times, when we are required to have our values not changed or altered throughout the program, we can create constants.
Q: How to create constants in C?
Ans: It is very easy to create constants in C. We just need to make use of the const keyword in C. Alternatively, we can also use the #define preprocessor directive to create constants.