In this tutorial, we are going to learn about comments in C, which is a very basic, yet very important concept to understand in C. Comments at times help us to understand the program through descriptions, or even in situations, when we want to avoid execution of certain lines of code, but want to keep them in our program.
We will see some examples, through which, we can understand the comments in C programming language. There are two types of comments that we have here – Single Line comments and Multiple line comments, and we will explore both in this tutorial.
Comments in C
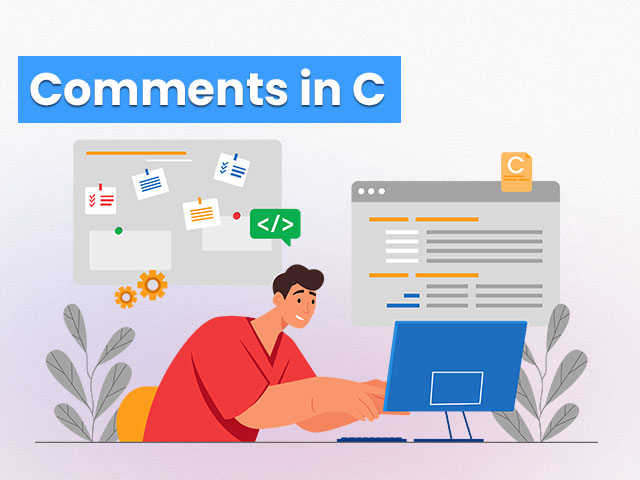
What is a comment?
Well, in our C programs, we often tend to use comments, in order to describe some particular instruction, some block of code, or the entire program. This makes our program more readable since we are actually describing what we are doing in the code. So, when our code is viewed by others, they can easily understand the program through the commented descriptions.
Comments are basically ignored by the compiler. So, we can also make use of the comments, in situations, when we need to have some particular code inside our program, but we do not want to execute it. So, if we comment on the code, just like any other comment, it would also be ignored.
So, enough of the explanation. Let’s now try to have a look at some programs, through which, we can understand how can we give some comments, and what are the different types of comments.
By the way, as it was stated earlier, we have two types of comments – single-line comments, and multiline comments.
Single-Line Comments in C
As the name says, with single-line comments, we get comments on a single line. So, whenever you want to make a one-liner comment, you can create a single-line comment, and write some description within that.
Giving a single-line comment is very simple. You just need to type //(two forward slashes), and anything that you write after these two forward slashes would be considered a comment(but only for the same line)
Single Line Comment in C Example
Let’s have a look at a simple example, which tries to demonstrate creating single-line comments.
#include <stdio.h>
int main()
{
// this is how you give some single line comment.
int age = 30; // this variable stores age.
printf(“You are %d years old!”, age);
return 0;
}
As you can see in the above program, we have given 2 single line comments here, which, one is just for demonstration, and the other comment, written just after we created the age variable and assigned value to it, is describing something about the variable that we have created. So, such single-line comments are at times useful, when we are required to describe something about some code.
Also, the comments can be used to avoid the execution of some instruction or block of code. The below example tries to demonstrate the same thing.
#include <stdio.h>
int main()
{
// this is how you give some single line comment.
int age = 30; // this variable stores age.
// printf(“You are %d years old!”, age);
return 0;
}
As you can see in the above example, the print f function has been commented, which means that just like any other comment, it would be also ignored by the compiler, and if you try to execute the program, you would not see any output on the console.
So, simply speaking, the single-line comments would be written in a single line. You can write single-line comments as and when required in your C programs.
Multiline Comments in C
After reading about the single-line comments, it becomes super easy to make a guess about the multiline comments. Using the multiline comments, you can write the comments, which can span multiple lines.
Writing multiline comments is also very easy. We just need to write /**/, and anything between /* and */ is going to be considered a multiline comment.
Multiline Comments in C Example
Let’s have a look at a simple example, through which, you can understand the concept and the need for multiline comments.
#include <stdio.h>
int main()
{
/*
This is a multiline comment,
which is why, it is spanning multiple lines.
You can still go on writing your description here.
*/
/*
In the below program, we are just
creating a variable to store age, and then we are
printing the age.
*/
int age = 30;
printf(“You are %d years old!”, age);
return 0;
}
As you can see in the above example, we have 2 multiline comments, out of which, the first one is just some demonstration, and the next comment is used to describe something about the program, in quite detail.
So, this is how you can simply use multiline comments if in case you are writing some description, which spans multiple lines.
But why do we write comments?
To answer this, you need to know what are comments. Well, the comment is a statement that is completely ignored by the compiler. So, if you want to write some description about a particular line of code, some function, or the entire program, then you can use the comments to do so. You can also use the comments to avoid some lines of code to execute but have it in the program.
Summary
In this tutorial, we learned about comments in C language. Comments are at times useful in our programs when we need to write some description about some line of code, some block of code, or the entire program. Comments can also be used to prevent some code from being executed, without being removed from the program.
We saw two types of comments in this tutorial – Single line comments, and multiline comments.
If you find this tutorial to be interesting, you can explore our other tutorials related to C programming language to learn more about C programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to comments in C
Q: What is a comment in C?
Ans: In C language, comments can be used to write some description about some code, some block of code, or the entire program. Comments can also be used to prevent some code from being executed, without being removed from the program.
Q: State different types of comments in C?
Ans: There are two types of comments in C – single line and multiline comments.
Q: How to give comments in C?
Ans: A single-line comment can just be written using //(double forward slashes), and a multiline comment can be given using /**/.