In this tutorial, we are going to learn about user input in C++. At times, in programming, taking data as user input is a lot more important. So, you should know about how can we take user input in C++. We will see some examples, which demonstrate how to take user input in C++.
When we leave the data input to the user, there is more flexibility, since the programmer does not need to hardcode the input values every time, and the user can conveniently put the required values. For example, if you visit an ATM machine, there you can input the amount that you want to withdraw, so you can see how important user input is in day-to-day life as well.
How to get user input in CPP?
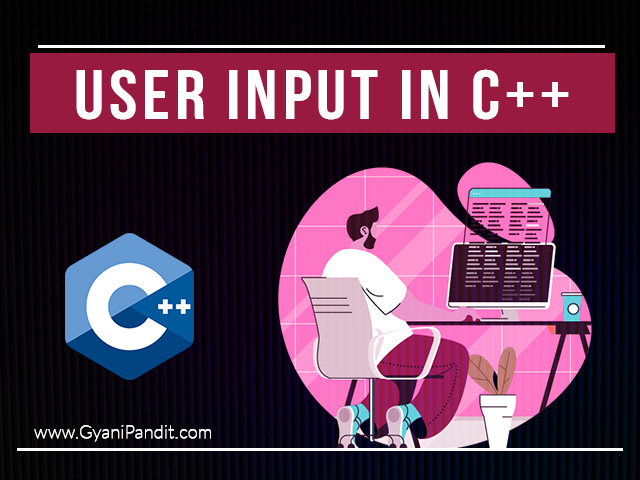
User inputs just mean that the data would be given to the program at the time of execution(most probably by the user). It is very easy to take user input in C++. you just have to the cin object to take the input. (cin means character input).
And when you get some input from the user, you need to store it somewhere, so there you will use the variables.
Let’s have a look at a simple program, which demonstrates using cin object, to get the user input. Remember that we are using cout just for showing some message so that the user can understand what he/she needs to input.
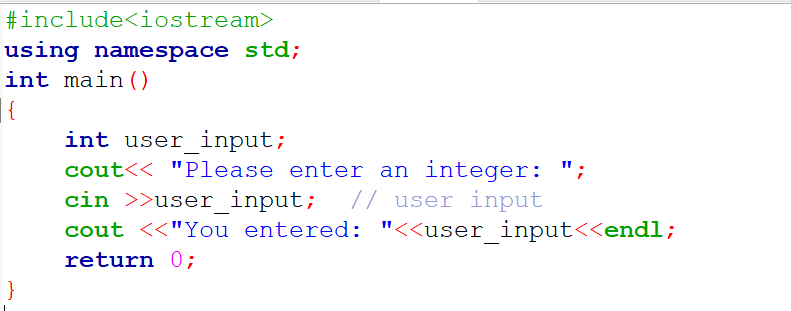
Let’s have a look at the output of the program –
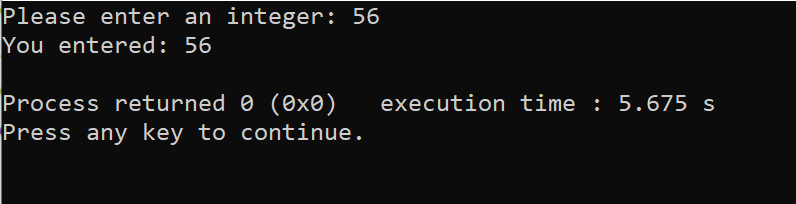
As you can see from the above program, we have used cin to get the user input. So, we have a variable with the name user_input, and then we are getting some integer as user input, and storing it in the variable.
Let’s have one more example, where we are getting some data as input from the user.
#include <iostream>int main()
{
int age;
std::cout << “Please enter your Age:”;
std::cin >> age;
std::cout << “You are “<<age << ” years old!”;
return 0;
}
Output –
Please enter your Age:30
You are 30 years old!
As you can see from the above program, we first get a message, the program seems to be waiting for some input, and then, when we input the age, we see some message as output. This is very simple and straightforward, and very important since you would be doing many programs in C++ ahead, and in many cases, you might need to get the data as user input.
Summary
In this tutorial, we learned about how can we take user input in C++. User input is an important thing in programming, and it is important for us to know how can we take data as user input in C++. We saw some examples, which demonstrate how cin object can be used for taking user input.
If you find this tutorial to be interesting, you can explore our other tutorials related to C programming language to learn more about C programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to user input in CPP
Q: How to take user input in C++?
Ans: To get user input in C++, we just need to make use of the cin object. You can refer to the above-stated programs, to understand how to use cin object, to get the user input.
Q: What is user input in C++?
Ans: In programming, user input means that the user is entering some data into the program, through some input means, rather than the programmer having to hard code it all the time.
Q: What is cin in C++?
Ans: We can simply understand that cin means character input in C++. The cin object belongs to the istream class. It is used when we need to take some input from the user in C++ programs.