While we are writing some programs in any programming language, we need to deal with data, and the data can be of some type, like int, float, char, etc. In any programming language, taking the data as user input can be crucial. For example, when you go to some ATM to transact money, how much money you want to withdraw is User Input in C, due to which, the user gets the flexibility.
So, in this article, we are going to learn about how can we take some data as user input in C programming, and it is very simple and very useful.
If you want to print something onto the screen, you make use of the printf function, right? In a similar way, if you want to take input from the user, you can make use of the scanf function.
User Input in C Programming
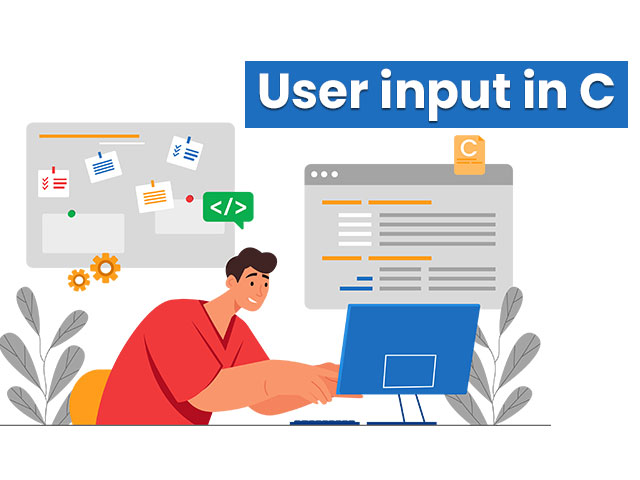
Table of Contents
So, here is a small demonstration of how you can take the user input and store it in some variable (of course the data will be of the same data type as the variable).
#include <stdio.h>
int main()
{
int age; // we will store the user input data in the variable.
printf(“Please enter your age:”);
scanf(“%d”, &age);
printf(“You are %d years old!”, age);
return 0;
}
As you can see in the above program, we have just created a variable with the name age, and we are going to get the age as user input. So, using the printf function, we are just giving some message, so that the user knows what to input, and then using the scanf function, we are getting the age of the person as user input. The &age that you can see in the program, just says that we are storing at the address of the variable.
The %d format specifier is being used here since we are taking the integer as user input. On the other hand, if you were taking float value as user input, the format specifier would be %f. So, you need to choose the format specifier accordingly.
Example of User Input in C Program
Let’s have a look at another example, using which, we would get a floating point number as user input.
#include <stdio.h>
int main()
{
float salary;
printf(“Please enter your salary:”);
scanf(“%f”, &salary);
printf(“You are %f years old!”, salary);
return 0;
}
So, as you can see in the above program, we have created a float type variable, and then, we are just taking the salary of the person as user input, and then we are just printing the salary.
Let’s have a look at another example, through which, we can understand getting the character input from the user.
#include <stdio.h>
int main()
{
char character;
printf(“Please enter some character:”);
scanf(“%c”, &character);
printf(“You entered %c!”, character);
return 0;
}
If you try to execute the above program, you can simply understand that we are getting a character input. For this, we are using the format specifier %c.
Format Specifiers in C
You have to be careful about the format specifiers, while you get the user input. Here, you can find the table, for format specifiers of different datatypes.
Data Type | Format Specifier |
---|---|
int | %d |
char | %c |
float | %f |
double | %lf |
short int | %hd |
unsigned int | %u |
long int | %li |
long long int | %lli |
unsigned long int | %lu |
unsigned long long int | %llu |
signed char | %c |
unsigned char | %c |
long double | %Lf |
Summary
In this tutorial, we learned about how can we take some data as input from the user. User input can prove to be an important step in any programming language, and in many programs.
So, now I hope that you have understood the following things →
- You use printf function to print something on the screen.
- You use scanf function to take user input.
- You have to use the format specifiers while you get the user input, and also when you output something on the screen.
If you find this tutorial interesting, you can learn more concepts related to C language with us on GyaniPandit, and also, you can explore a lot of other courses, related to Python, Machine learning, and Data Science on GyaniPandit, and upskill yourself.
FAQs related to User Input in C Programming
Q: How to take user input in C?
Ans: To get the user input in C language, we can make use of the scanf function.
Q: What is %d in C?
Ans: %d is a format specifier used for integer datatype.
Q: What is the use of scanf in C?
Ans: The scanf function commonly is used for taking input from the user in the program.