TYPECASTING (CONVERTING ONE TYPE INTO ANOTHER)
Since we have to deal with different types of data in python, we are having some different datatypes. But at times, there might be a requirement to change the type of data, from one type to another. This thing is called typecasting. We are going to have a look at how we are going to achieve this, and we would also have a look at some of the examples, to get a clearer idea about the typecasting.
Typecasting Python
While we explore the typecasting, we would go through some different types of typecasting, like-
- Implicit Typecasting
- Explicit Typecasting
IMPLICIT TYPECASTING IN PYTHON
Well, by typecasting, we mean the conversion of data from one type to another. When the type of the typecasting is implicit, we do not have to do anything, and the typecasting is done automatically. Let’s have an example so that we can understand what is happening in the implicit typecasting. Just understand that we do not need to do anything, and it happens automatically.

As you can see in the above program, we have created two variables, where one has the int type data, and the another has float type data. Now, we are trying to add the two numbers, and what would be the type of the result? Well, it is going to be the float type.
So, the type of the new data becomes float, and we had to do nothing. This is the implicit typecasting thing. On the other hand, there is something else, called as Explicit Typecasting. Now, let’s explore the concept of explicit typecasting.
EXPLICIT TYPECASTING IN PYTHON
As the name says, explicit typecasting would be in some situations, when we are required to do the typecasting. So, this time, it is not going to happen on it’s own, but we have to explicitly do it. But how are we going to do this thing explicitly? Do we have something? The answer to this question is YES! We have got some functions, so that we can do the typecasting. Later, we are going to have a look at some examples, which would make the thing clear, that why would we ever need to do the typecasting?
Here is a list of some of the functions that are mainly used for typecasting –
Function | Description | Example |
int(x) | This takes the float value or the string value as an argument and returns int type object. | If x = ‘3’, which is a string, then int(x) makes it as an integer. Even x = 3.5, which is a fractional number, can be converted to an integer using the same function. |
float(x) | This takes the int or string value as an argument and returns a float type object. | If x = ‘3’, which is a string, then float(x) converts it to a floating-point value. Similarly, a value x=4 can be converted to float. |
str(x) | This takes the int or float value as an argument and returns a string type object. | If x = 5, which is an integer, then str(x) returns a string object for this. |
There are some more functions as well, like there is bool function, which is used to return the boolean value of the given object. Now, Let’s have a look at an example to understand the concept of the explicit typecasting. Let’s have a look at an example, where we would use the above stated functions, for typecasting –
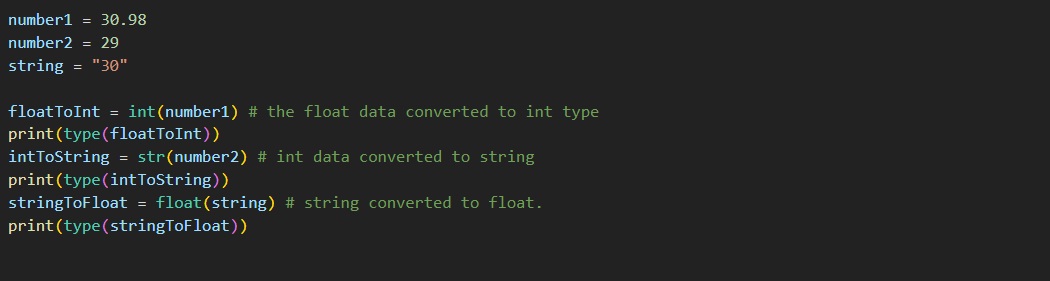
As you can see, we are explicitly converting the types of the data, from one type to another(of course the data should be valid to do so).
Now, the thing is that where we would want to use this? Why would we require to do explicit typecasting? Well, the answer lies in the below use case.
Let’s consider a situation, where we are required to take the data input from the user. In such cases, we would use something called as an input function in python. The input function is used for taking the user input. The thing is that the input function is always going to return the string object, even if the user enters some int data, or even float data. So, this means that the user input is always going to be a string, and we are required to convert the data according to what we are required, like int, or float for example.
Let’s have a look at the code now –

As you can see, in the above program, we are using the input function, to take the user input, and the data that we are going to get, is assigned to the reference variable number. The thing is that, we are asking the user for number, and the user is most probably going to enter some number only. But the input function is going to return a string type object. You can try executing the above program and you would find the type of the object to be string. But, if we need an integer over here, we have to typecast the input data, into the int type.

Now, we know that the int function can be used for converting the string or float data to int, we are going to wrap the input function, into the int function, so that the string type object returned by the input function would be converted to int type.
Now, we have explicitly converted the string that is being returned from the input function to an int type data. You can now try executing the above program and have a look at the output, and you would see that the type of the object is now int.
One more thing to notice, is that we cannot convert any sort of data to any other datatype. The type needs to be convertible. For example, the below thing is not going to work.

As you can see, in the above program, we are trying to perform an invalid datatype conversion operation here, so we are going to get a ValueError, for not giving the valid type data for conversion.
So, you also have to keep in mind, that even if we are doing the datatype conversion, it is necessary to know that the type of the data should also be valid for conversion.
There are some other functions as well, like if we want to convert something to list, tuple, set, etc.., then we have some functions for that, but we shall make use of them, as and when required.