Syntax in C
In this article, we are going to learn about Syntax in C, and we will also try to perform a basic C program, so as to understand the flow and get hands-on experience doing our very first program in C.
By syntax, we mean that we are trying to define a set of rules for defining the structure of some language. Since from now, you will be writing a lot of programs in C programming language, you should be familiar with the basic syntax, so that you can easily use the things later. Also, we are going to perform a simple program, so as to understand the different things in the program.
Syntax in C and Basic Program
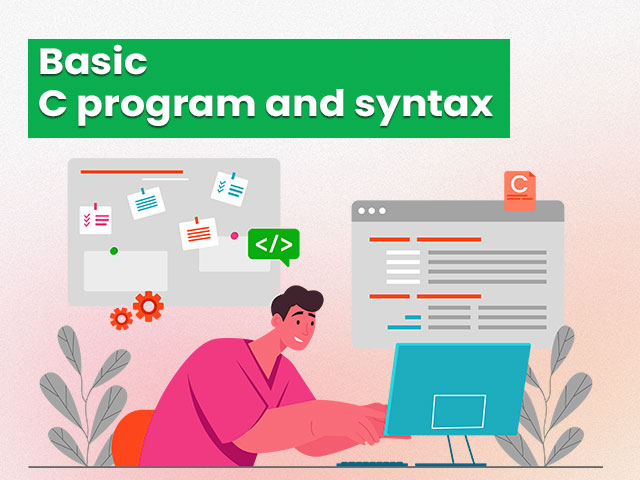
Here is the basic boilerplate for a C program. By boilerplate, we mean that these are some things, that you would see mostly in your C programs.
#include <stdio.h>
int main() {
// Your code here
return 0;
}
As you can see here, we have some simple lines of code, and now, let’s understand them one by one so that later on, you can feel comfortable using these things in your programs.
These are some of the things that you can observe in the above code –
- #include<stdio.h>
- int main()
- //your code here
- return 0;
(And do not forget about the brackets. These are also very important)
There can be a lot of other things in your programs, but these can be considered some of the basic things that you would see in many C programs. So, now let’s have a look at them one by one.
- So, here, the first line, in which we wrote something like #include<stdio.h>(this is called preprocessor directive), is saying that we are including something(some header file. A header file is just a file with some function declarations, which can be used in this particular program). Here, stdio.h is a header file(a header file has a .h extension).
- In the next line, the main function is the entry point of your program.
- Within the two curly brackets after the main, we are going to write our code(logic), as we are going to see ahead through a simple example.
- The “//your code here” is just a comment here(actually a single-line comment). Well, you would later study comments, but you can consider for now that comments are used to describe some block of code. It is basically ignored by the compiler.
- In the next line, there is a return 0; This means that the program was executed successfully.
The C program stops execution, when there is no program statement to be executed. Later on, when we would see an example, you would observe that for the termination of some instruction, we need to use a semicolon here. You can understand this as if in order to finish some English sentence, we need to use a full stop, just like that, here, in our C programs, we use semicolons.
Let’s have a look at a simple C program, where we are going to print a simple message, like Hello World or something else.
#include <stdio.h>
int main()
{
printf(“Hello, World!n”);
printf(“Hello From GyaniPandit”);
return 0;
}
As you can see in the above program, we have written two very simple printf functions, and as the name suggests, this function is used for output purposes. As you would move ahead, learning more about C programming language, you would simply understand the concept and would be able to use it further.
So, using the first printf function, we are able to print the string Hello World! As an output, and the ‘n’ here is given for a new line, and so on the next line, we get output as Hello From GyaniPandit.
Also, notice that we have semicolons used for marking the end of the statement.
Here is the output of the above simple program –
Hello World
Hello From GyaniPandit
Later on, when you would write some more C programs, you would get comfortable with the syntax, but here, it was just an acquaintance with the different things that are going to be there in our C programs.
Summary
In this article, we have seen the syntax in C program, and also a very basic C program, through which, we learned a very basic operation of doing some output. Later on, as you do more and more C programs, you would get familiar with the syntax, and other concepts, but still, this can be considered as a brief introduction to the different things that are there in C programs, and remember that there is a lot to learn and understand ahead.
FAQs related to Syntax in C and Basic Program
Q: Why do we use a semicolon in C?
Ans: The semicolon is basically used to mark the end of some statement. You can consider some statements to be a block of code, which performs some action. You can understand when we are writing some sentence in English, and in order to complete that, we need a full stop, so similarly here, we are using the semicolon.
Q: Is the C program hard to write?
Ans: No, C programs are neither hard, nor easy to write, but it depends from person to person. Someone may find it very easy, and on the other hand, somebody else would find it harder. So, with practice, and a good understanding of the concepts, you can easily write C programs. You can follow our detailed C language tutorials, to learn C programming language. Also, you can explore many other courses, related to Python, Machine learning, and Data Science to upskill yourself.