In this tutorial, we are going to understand what is shadowing in JavaScript. But in order to understand shadowing in JavaScript, one needs to be familiar with the concept of scope in programming. So, for the sake of better understanding the concept of shadowing, we would also cover some brief about the scope.
Shadowing JavaScript
Well, shadowing is a pretty interesting and simple concept, and not knowing about shadowing may cause misconceptions at times.
Now, let’s consider a program, and then later, we will try to explain what the output is –

If we try to run the program, what is going to be the output? Well, I should not even ask this. You know that the output is going to be 10 right? But let’s say… what about this one? Have a look –

Well, now also, the output is going to be 10 simply. But how about this?

Now, the output is going to be 100. but why am I even asking all this? Well, the mystery lies in the above program. Let’s just modify the above program a little bit now.
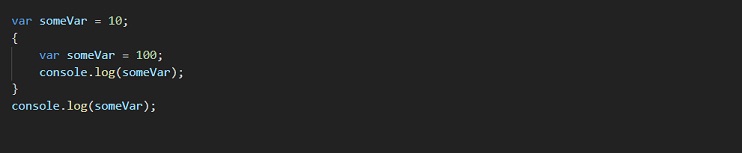
As you can see, we are trying to log some Var to the console, inside the block once, and outside the block afterward. If we try to run the above program, the output comes out to be –
100
100
Well, to understand this behavior, we need to understand the concept of shadowing, and here, we are going to understand the concept of shadowing, with the help of suitable examples, so that it becomes easy to understand.
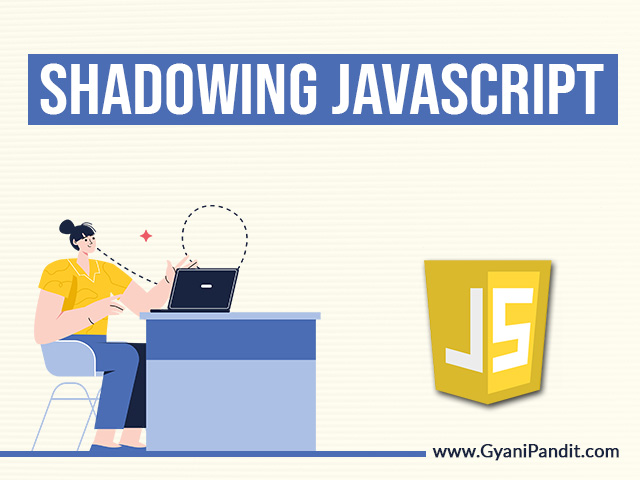
What is shadowing in JavaScript?
In order to understand shadowing, we need to understand what is scope first. In programming, we can understand scope as the region of the program, where a defined variable is recognized and can be accessed. Beyond that scope, it cannot be accessed. There can be different kinds of scopes in the context of JavaScript, but here, we would focus on a global scope and block scope.
So, with block scope, we can understand that the scope of the variable is limited to the block. So, the variable cannot be accessed outside the block. When we would have a look at some examples, you would comfortably understand the concept. When we create variables with the keywords let and const, they are block scoped. Have a look at the below example, through which, you would understand the block scope –
{
let x = 100;
// inside the block, we are able to access the block.
console.log(x);
}
// outside that block, we cannot access the variable x.
console.log(x)
As you can see, we have a block in the above program. To create a block, we simply need to make use of the curly braces {}. The block is basically used to combine multiple statements. In the above program, you can see that inside the block, we have a variable that is created using the let keyword. So, this variable is block scoped, which means that this variable can be accessed only in this block, and not outside.
So, if we are accessing the element inside that block(same scope), then it is fine, but when we try accessing the same variable outside the block, we would not be able to do so, since we cannot access it beyond the scope. You can try executing the above program, and find that we are getting errors when we are trying to access the variable x outside the block.
On the other hand, we have something called a global scope, which basically means that we can use this variable anywhere in our JavaScript program. When we create a variable using the var keyword, outside any function, that variable has a global scope. Or if the variable created with the var keyword is inside some function, then it has a function scope.
Let’s have a look at another program, through which, we can understand about global scope in JavaScript.
var number = 100;
{
// the blocks do not scope the var declaration.
var anotherNumber = 200;
console.log(“anotherNumber:”, anotherNumber);
// the variable created with var can be used here.
console.log(“number:”, number)
}
// we are able to access this variable outside the block as well.
console.log(“anotherNumber:”, anotherNumber);
As you can see in the above program, we have created variables with the var keyword, both inside the block, and outside the block. The var keyword, when created outside the function, has a global scope.
So, we can access those variables from anywhere in the program. You can try executing the above program, and find that you can use the variables made with the var keyword, anywhere in the program.
But what is shadowing in JavaScript?
Well, we hope that you have understood the scope of variables in JavaScript, and till now, we have not discussed anything related to shadowing in JavaScript, except that we stated above that we are going to discuss shadowing here. So, now, after understanding the concept of the scope of variables, we are now going to understand what is shadowing.
The variable is said to be shadowed when the variable is declared in the outer scope, and the variable with the same name is declared in the inner scope, the variable in the inner scope can be said to shadow the variable in the outer scope. Have a look at the below example, through which, we can get an initial idea about what is happening.
var number = 100
{
var number = 200
console.log(number);
}
console.log(number);
As you can see in the above program, we have the variable named “number” created with the var keyword. Then, we have a simple block, in which, we again have the variable made with the var keyword, named “number”. Now here, we can say that the variable in the inner scope has shadowed the variable from the outer scope. The thing is that in this case, the value for the number variable also becomes 200. If you look at the output, you can find that we get output as 200 in both cases.
But this is not the same case with the variable made with the let keyword. This is because of scope.
Let’s have a look at the program, which makes us familiar with the case with the let keyword.
let number = 100
{
let number = 200
console.log(number);
}
console.log(number);
As you can see in the above program, we have created a variable with help of the let keyword, outside the block, and inside the block as well. So here, the variable from the inner scope is said to shadow the variable from the outer scope. In this case, if you execute the above program, you can see the output as first 200, and then 100.
Also, we can have var in the outer scope, and let in the inner scope. But the vice versa won’t be true, since it leads to illegal shadowing, which we will discuss soon, but first let’s have a look at an example, to get familiar with teh concept of shadowing.
var number = 100
{
let number = 200
console.log(number);
}
console.log(number);
In this above example as well, the output comes out to be 200 and 100 only. But as said earlier, the vice versa won’t be true, which means that we cannot let in the outer scope and var in the inner scope. This would lead to something called illegal shadowing. So, let’s discuss this.
What is Illegal Shadowing?
With shadowing, we can say that we have a variable in the outer scope, and we have another variable with the same name in the inner scope. So, the variable with the inner scope shadows the variable with the outer scope. But here, one thing is to be noticed, when shadowing a variable, it should not cross the scope boundary. So, if we are writing a variable with the let keyword in the outer scope, and with the var keyword in the inner scope, this won’t work, and we would get an error saying that the variable has already been declared.
Let’s try to have a look at a simple program, through which, we can understand the idea.
let number = 100
{
var number = 200
console.log(number);
}
console.log(number);
If you try to execute the above program, you would get an error, saying that the variable is already declared. So, we can shadow a var variable, with the let variable, but the opposite is not true.
Conclusion:
In this article, we tried to understand the concept of shadowing, through some related examples. We hope that you could understand the concept, along with the examples. You can try the above examples, in order to have a look at them, and understand them. But one should avoid giving the same names to the variables in certain different scopes, since some problems may arise in the code due to this.
Frequently asked questions related to Shadowing in JavaScript
Q: What is the scope of the variable?
Ans: The scope of the variable can be understood as the region, where that variable can be accessed, and not outside that.
Q: What is illegal shadowing?
Ans: Illegal shadowing is if while shadowing a variable, it crosses the boundary of the scope. So, while shadowing, the scope boundary should also be taken into account.
Q: How to create a block in JavaScript?
Ans: To create a block in JavaScript, we just need to make use of the curly braces.
Q: What is block scope in JavaScript?
Ans: With block scope, we mean that we can only access the variable inside the particular block only. When the variables are created with let and const keywords, they are block scoped.
Q: What is the global scope of JavaScript?
Ans: With the global scope, we mean that the variable with a global scope would be accessible anywhere in the program. When we use the var keyword to create a variable, outside the function, it is in the global scope.