Convert Celsius to Fahrenheit in Python
In this article, we are going to write a simple Python program, to convert the temperature from degree Celsius to degrees Fahrenheit. This program is going to be a simple formula-based program, in which, we just have to take the temperature in degrees Celsius as user input, and then we have to apply the formula and show the output to the user.
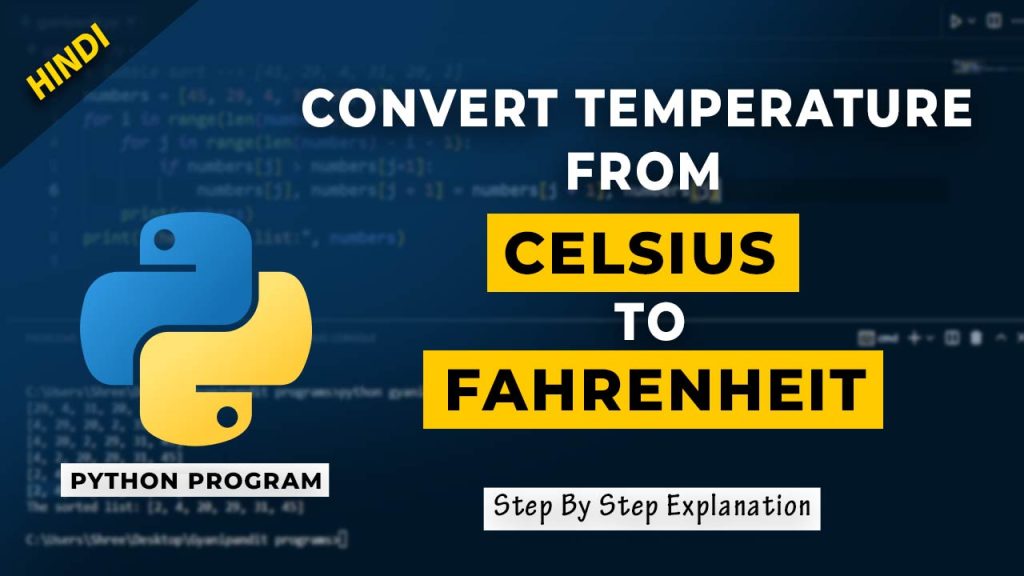
So, this is the formula for converting temperature from degree Celsius to degree Fahrenheit –
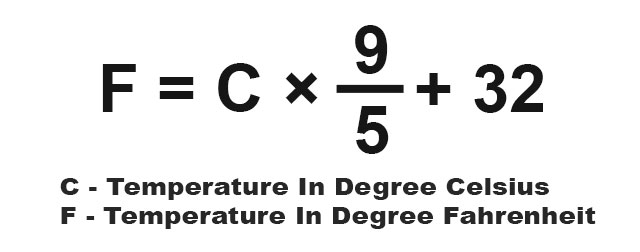
So now, let’s try to have a look at this simple program, through which, we would try to convert the temperature from degree Celsius to degrees Fahrenheit.
Python Program to Convert Celsius to Fahrenheit
celsius = float(input(“Please enter the temperature in celsius:”))
fahrenheit = (celsius * 9/5) + 32
print(f”{celsius} degree Celsius is {fahrenheit} degree Fahrenheit.”)
As you can see in the above program, we are getting the temperature in degrees Celsius as user input. After that, we are just applying the formula to convert the temperature from degree Celsius to degree Fahrenheit. After that, we are showing the temperature in degrees Fahrenheit as output.
You can try executing the above program and observing the output for different values. Let’s have a look at the output for the above program –
Output 1 –
Please enter the temperature in celsius:35
35.0 degree Celsius is 95.0 degrees Fahrenheit.
Output 2 –
Please enter the temperature in celsius:46
46.0 degree Celsius is 114.8 degrees Fahrenheit.
As you can see from the output, we have to input the temperature in degrees Celsius, and as an output, we get the temperature in degrees Fahrenheit.
Python Program to Convert Celsius to Fahrenheit step by step
Now, when we are done with the program, and the input-output, let’s now understand the program step by step.
- We are taking the temperature in degrees Celsius as user input
- When we have the temperature in degrees Celsius, we just apply the formula to convert the temperature from degree Celsius to degrees Fahrenheit, and we have a reference variable Fahrenheit for the temperature in degrees Fahrenheit.
- After that, we show the temperature in degrees Fahrenheit as the output of the program.
Conclusion
We have understood and implemented a simple python program, to convert temperature from degree Celsius to degrees Fahrenheit. We hope that you have understood the program, and you can try many such other programs.
FAQ Python Program to Convert Celsius to Fahrenheit
Q: How much is 32 degree Celsius in degrees Fahrenheit?
Ans: 32 degrees Celsius is 89.6 degrees Fahrenheit.Â
Q: How to convert temperature from degree Celsius to degrees Fahrenheit?
Ans: To convert temperature from degree Celsius to degrees Fahrenheit, you can use a simple conversion formula, which is f = (c * 9/5) + 32, where c is the temperature in degrees Celsius, and f is the temperature in degrees Fahrenheit.
Q: How to convert temperature from degrees Fahrenheit to degrees Celsius?
Ans: To convert temperature from degrees Fahrenheit to degree Celsius, you can use a simple conversion formula, which is c = (f -32) * 5/9, where f is the temperature in degrees Fahrenheit, and c is the temperature in degrees Celsius.