You might be familiar with the concept of operators in C. So now, in this tutorial, we are going to learn about operator precedence and associativity. The concept is very simple, but not understanding it, can lead to further confusion when you would actually use different operators in your expressions.
Operator Precedence and Associativity in C
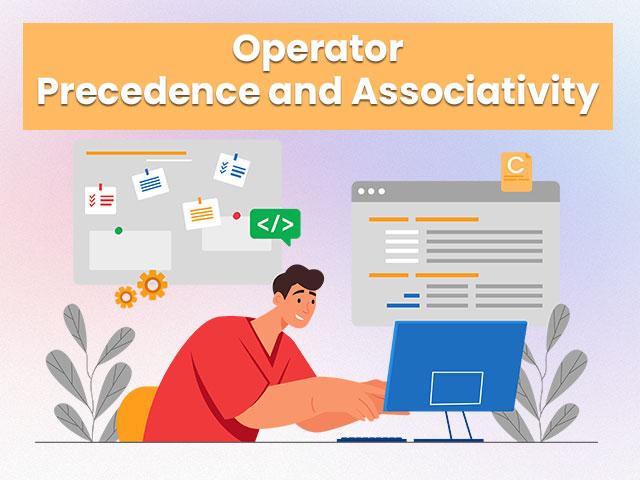
What is Operator Precedence in C
When you are using some operator in an expression, there is no problem if there is only one operator. But there might be situations when there can be two or more operators in one expression and at this time you could be confused regarding which operator will be executed first.
Let’s consider an example,
#include<stdio.h>
int main()
{
int expression ;
expression = 7*9-2;
printf(“ %d ” , expression);
return 0;
}
In the above example, you can see that the expression has an operator for multiplication, as well as subtraction. So, now the confusion is how can we decide which operation should be done first. Should it be multiplication, or should it be subtraction, or should we go left to right, or right to left, or should we go in the alphabetical order for the operation?
This is where the operator precedence comes into the picture. We follow Operator Precedence in C programming language, to get the priority of execution of the operations.
So what operator precedence is going to do is, it is going tell us regarding the priority of execution of these operators in the expression. What priority should be given to different operators when there are multiple operators in one expression to evaluate? Now the priority is nothing but deciding which operator should be executed first and which should be executed after that and so on.
As we had seen in Mathematics a BODMAS rule is applied to solve certain mathematical expressions so here in C programming as well we have Operator Precedence to be followed.
You do not have to remember the order of precedence, but just remember that there is something that decides how the operations are going to be executed. You can below find a table for a reference of operator precedence.
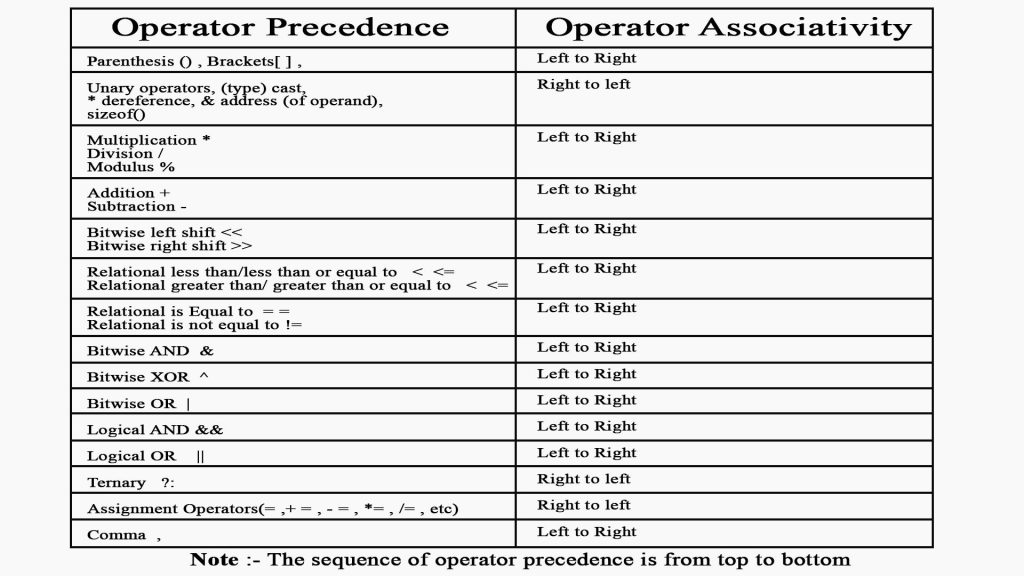
Given below are many different operators in the C programming language and they are arranged according to their precedence from top to bottom this implies that the topmost operator mentioned in the chart will have the highest precedence that is the highest priority and the bottommost operator is going to have the least precedence and along with this the associativity of these operators is also mentioned next to them.
So, from the previous program, the multiplication operation is done first, since multiplication has greater precedence than that subtraction. You can look for the precedence table, to understand the order of precedence for different operators. So, the output of the above program would be 61.
What is Operator Associativity in C
Now, you might be familiar with the concept of operator precedence. But if you have observed the table, you might have seen that some operations have the same precedence, like multiplication and division, addition and subtraction, and they have the same level of precedence. So, how about if they are both used in the same expression? Now again the question is what will execute first?
So, this is where the operator associativity comes into the picture. So, let’s understand the concept of operator Associativity.
Operator associativity is going to determine the order of the operations to get executed when in an expression there are operators of the same precedence.
So now to execute them the order can either be from left to right or from right to left. In most of the cases, the associativity is from left to right, as you can see from the given reference table.
Now following that table, we can easily solve many mathematical expressions here in C programming which contains many different operators in it all together.
Now again if we talk about the same example that we were discussing earlier so the correct answer to that particular example is going to be 61. You can have a look again.
#include<stdio.h>
int main()
{
int expression ;
expression = 7*9-2;
printf(“ %d ” , expression);
return 0;
}
So here as the multiplication operator has higher precedence than the subtraction operator we can imply this from the table given above, so the multiplication operation will execute first and then be followed by the subtraction.
Let’s consider one more example right here, in which, we would have some operators, with the same level of precedence. Also, since we are using the brackets here, they have higher precedence.
#include<stdio.h>
int main()
{
int expression ;
expression = 50/5*2+(17-9);
printf(“ %d ” , expression);
return 0;
}
So now here in this expression, you can see that there are some operators of the same precedence as well so here operator associativity will also be used, and keeping in mind both of them the given expression will be solved, So give it a try yourself.
The correct answer to this expression is going to be 28 by following the operator precedence and associativity.
Summary
In this tutorial, we have learned about Operator Precedence and Associativity in the case of the C programming language. This concept is very simple and easy to understand, but at the same time, it can confuse a lot, if not done properly.
With operator precedence, we can decide the order of execution of the operations, when we have multiple operators. On the other hand, operator associativity comes into the picture, when we have operators with the same associativity in the expression.
If you find this tutorial to be interesting, you can explore our other tutorials related to C programming language to learn more about C programming language. You can also explore our other courses, related to Python, Machine learning, and Data Science, through which, you can upskill yourself.
FAQs related to Operator Precedence and Associativity in C
Q: What is Operator Precedence in C?
Ans: With operator precedence, we can decide the order, in which the operations would be performed in an expression when we have multiple operators used in an expression.
Q: What is Operator Associativity in C?
Ans: Operator Associativity comes into the picture when we have multiple operators with the same precedence, being used in the expression.