Operator overloading in Python
We have come across many different operators at times. We use them for performing some different operations. Like if we consider the + operator, we get to see that the + operator is basically used to add the numbers. Also, if we are using the + operator with the strings, then it is used for string concatenation, and if we are using the + operator with lists, we can use it to merge the lists. But how? how is the same operator behaving differently for different types of objects, like for strings, integers, and lists as well? Well, this is because the meaning of the + operator is extended beyond its predefined operation.
This is referred to as operator overloading. This simply means extending the meaning of the particular operator, to use it for something else, other than its predefined meaning. From the above example, the + operator is overloaded, due to which, we can use it for different things. When the same operator is showing different behavior with different objects, it can be referred to as Operator Overloading.
Operator Overloading in Python
Now, Let’s put light on how this happens, and how we can do it. The thing is that when we use an operator, automatically, a magic method gets called, which is associated with that operator. For example, when we are using the + operator, automatically the magic method __add__ gets called. So, if we want to perform operator overloading, we just need to define the __add__ method in a way related to what we want to do, and the operator would behave in that way.
As an example of the magic method, Let’s consider using the __add__ method and adding the two integers.

If you try to run the above program, you will get the same output in both cases. So, for the different operators, we have some different magic methods, for multiplication, we have the __mul__ method, and for subtraction, we have the __sub__ method, and so on. To understand the concept in a better way, we would have a look at a simple example. Just understand that we need to define the magic method associated with the particular operator, and then the operator would behave in that way.
Let’s consider an example. Let’s say that we have a class called Book. Now, we have two Book objects with us, and we wish to add them, so we would simply use the + operator to do so. But in the below example, we do not have defined the __add__ method, so we are going to have an error here. Have a look at the below example, which gives us the same idea.
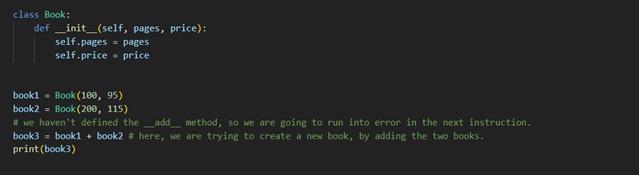
As you can see, in the below program, we have a class Book, in which, we have the special __init__ method. When we are creating the Book object, we are passing the number of pages, and the price of the book as arguments. After that, we are trying to use the + operator, to add the two books. The simple thing that we want to do here, is that we want to add the two books and create a new book, which has the pages from both books. This is like you took two books, and merged them into one, so the page number is now increased, and the price too, to some extent. So, this example makes some sense.
But, in this case, we are not going to be able to do these things, since when some operator is used, automatically, the magic method associated with it executes. But here, for the books, we have not defined the magic method, so we are going to run into an error. If you try to run the above program, we are going to get the error, which is something like this –
Traceback (most recent call last):
File “C:\Users\Administrator\Desktop\programs\duck.py”, line 232, in <module>
book3 = book1 + book2 # here, we are trying to create a new book, by adding the two books.
TypeError: unsupported operand type(s) for +: ‘Book’ and ‘Book’
As you can see, we got the TypeError, saying unsupported operand types for +. This simply means that it does not know what to do when the + operator is used on two Book objects. So, we need to define the special method or the magic method here, which is __add__. Let’s do it now.
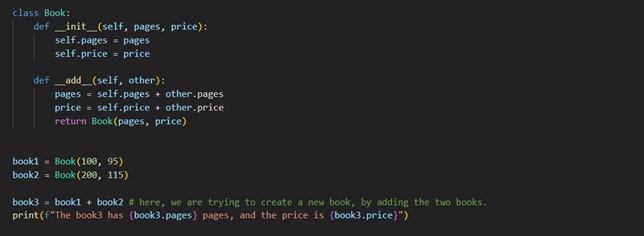
As you can see, now, the special method __add__ is now defined, and we are doing it in a way, that we are accepting both the objects, adding their pages and price, and then creating a new Book object, with that pages and price. Remember that the self is one object and the other is another object here in this case.
In this way, we have overloaded the + operator, to use it with the Book objects. You can also try doing this for some different examples as well, so that you can get familiar with the concept, and use it as and when needed.