Multiple inheritances in Python
When it comes to multiple inheritances, it simply means that one child class is inheriting from more than one parent class. So, for one child class, there are going to be multiple parents. Now, we are going to have a look at an example, through which, we would understand how we can do this.
As a simple example to understand, we would consider that there are two parent classes, Parent1, and Parent2, and there is one child class, which inherits from both the parent classes. Let’s have a look at this
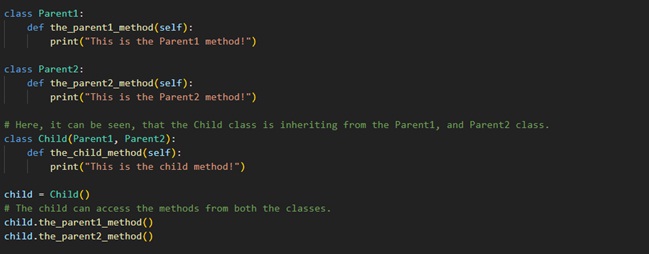
Multiple inheritances in Python
As you can see in the above program, we have tried to implement multiple inheritances. In the brackets where we write the superclass, you have to write the classes with commas separated. So, here, we can say that the Child class inherits from the Parent1 and Parent2 classes.
We know that the child class object can access both methods from different parent classes. So, what if both the parent class have methods with the same name? If we call the method from the child class object, then what is going to happen?
Let’s have a look at the next example, which tries to question the same thing –
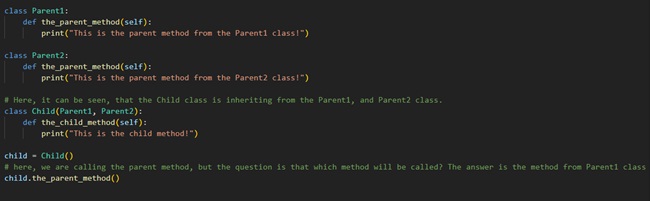
As can be seen in the above program, both the parent classes have methods with the same names, and then the child class object is calling that method. Now, the question is that which method would be called? Would it be from the Parent1 class, or from the Parent2 class? Well, you might have read the answer already, which is from Parent1(if you have not read the answer, read the comments of the above program again). But now, Let’s try to explain why is this happening.
The thing is that there is something called a Method resolution order. We can simply understand it as if we are calling some method, in what order will it be searched in the classes.
So, very first, if we are calling the method, it will be searched in the current class itself, and if not found, it will be looked for in the parent classes, from left to right(in our case, if the method is not in the child class, then it will be looked in the Parent1 class, and then in the Parent2 class, and finally in the object class).
So, you can try removing the method from the Parent1 class from the previous program and if you run the program again, you can find that the method from the Parent2 class is called.
Now, the question comes that we can have a look at this order. Well, the answer is an absolute YES. For doing this, we are going to use the mro method (or we can also use the __mro__ attribute), to get the order. Let’s have a look at the program which shows the same thing –
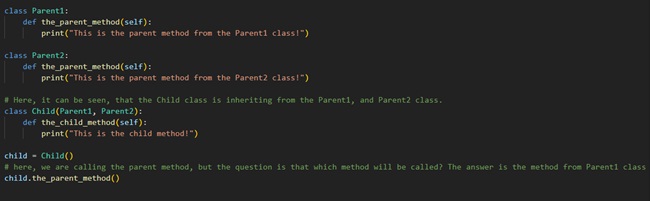
As you can see, we are using the mro method on the Child class. The method returns the method resolution order for the class, which is a list. Have a look at the output now –
[<class ‘__main__.Child’>, <class ‘__main__.Parent1’>, <class ‘__main__.Parent2’>, <class ‘object’>]
This is the parent method from the Parent1 class!
As you can see in the output, the method resolution order can be seen. Firstly, it will look at the Child class, then Parent1, then Parent2, and then the object class. This is very important for us, to understand the concept of what is going to happen so that we can avoid unexpected things happening in our programs.
You can also refer to the diagram, which demonstrates multiple inheritances.
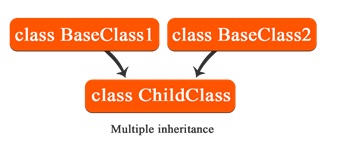
Now, Let’s turn to discuss another type of inheritance, which is hierarchical inheritance.