Multidimensional Arrays Java
Well, we now know that arrays are a collection of data of similar data types. Till now, what we were creating was a 1-dimensional array, which basically means that we are creating an array that stores some elements, like integers, characters, strings, etc.
But now, we are going to dive in and learn about multi-dimensional arrays. A multi-dimensional array can be considered an array of arrays. This means that this time, we are going to create an array, that has more arrays as its elements. This can be further expanded. Basically, we would be dealing with two-dimensional arrays at an initial level, but it can be taken much further as required.
Introduction to 2-dimensional arrays – 2D Array Java
So, what is a two-dimensional array, or a 2-D array? Well, when we were dealing with a one-dimensional array(or 1 – D array), each array element was just an element, like some integer, some string object, etc. But here, in a two-dimensional array, each element of an array is going to be an array. Let’s understand this concept bit by bit.
If you are familiar with the concept of the matrix from mathematics, we can consider a 2D (2 dimensional) array as a matrix, or some table with rows and columns, in which we add the elements in row-wise. We are going to see how can we take input into the 2D array. First, let’s see the syntax, like how are we going to create a 2D array –
type[][] arrayname = new type[rowsize][colsize];
The type refers to the type of data that your array is going to hold. This is like if the array is going to hold all the integer arrays, then the type of the main array would also be int.
With the two pairs of square brackets, we are telling that this is going to be a two-dimensional array. After this, we are given the name of the reference variable which will be pointing to the array object.
On the other side of the assignment operator, there is the new keyword, which is used to create an object. We are then again writing the same type there, and then again two pairs of square brackets. This time, we are specifying the number of rows in the first bracket, and the number of columns in the next bracket.
Well, adding the number of rows is compulsory, while adding a number of columns is not compulsory. We will deal with why mentioning the number of columns is not necessary.
Here is an example of how are we going to create a 2 dimensional array –
int[][] arr = new int[2][2];
Here, in the above instruction, we have created an integer 2 Dimensional array with a reference variable arr. This is going to have 2 rows and 2 columns as specified at the time of creation. You can also skip adding the number of columns. We are going to see ahead why is it not compulsory to add a number of columns.
First, let’s visualize what is happening here when we are executing this particular instruction –
int[] arr = new int[6];
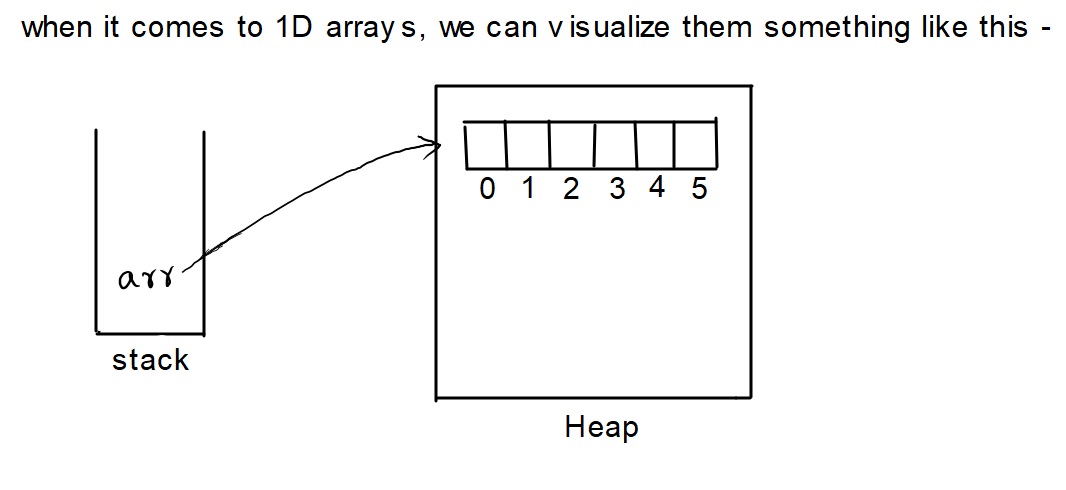
So, the arr is the reference variable in the above diagram, and it is pointing to an array. Also, one thing to note is that the array is going to contain integers in the above examples. But, when we are dealing with multidimensional arrays, the element inside the array is also an array.
In short, there would be some reference variable pointing towards an array, and then that array contains more reference variables that point to the array again. This is a chain here. In the case of a 2D array, we can consider that there is a reference variable pointing towards an array, and each element of that array is an array again. So, in short, every element of the array is another reference variable pointing to another array in the heap. Let’s visualize this thing to have a clear picture.
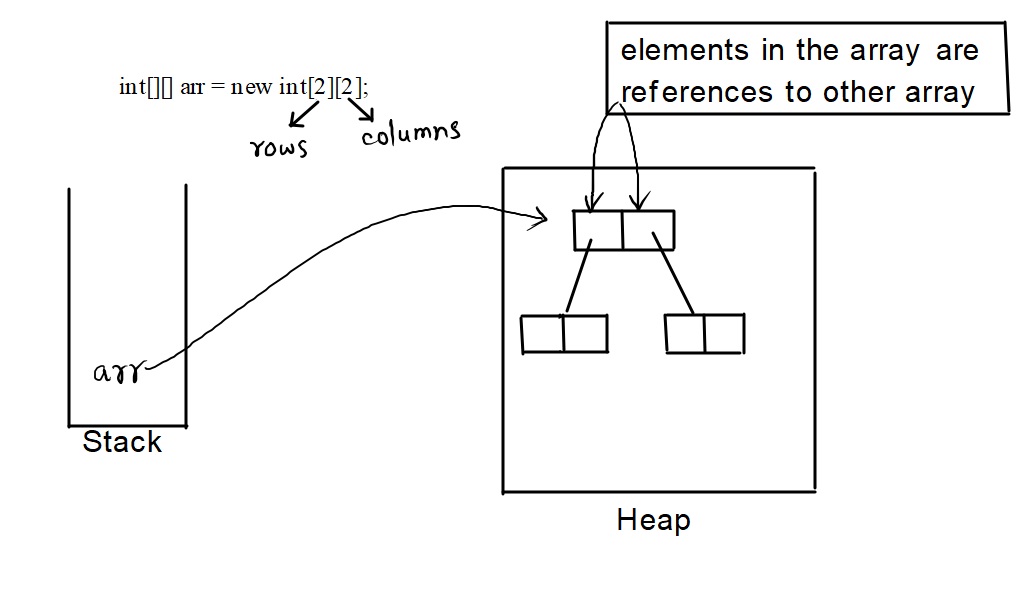
The above diagram is an example illustration of how 2D arrays are implemented. Let’s also consider another diagram through which we can understand how the elements are added, and also let’s understand the indexes.
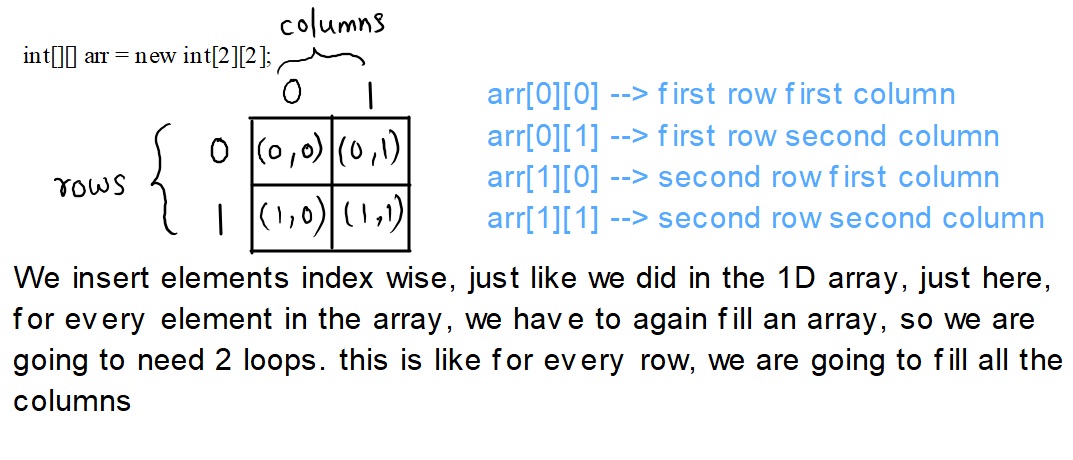
Earlier, just by using the indexes, we could access the array elements. But here, every element of the array is an array itself.
So, when we do arr[0] for example, we get another array. Again if we do arr[0][0], then we are getting the first element of that first array. If we do arr[0][1], it gives the second element of the first array.
If this confuses you, lets have an example program in which we will learn how to give inputs to this array.
import java.util.Arrays;
import java.util.Scanner;
public class ArrayBasic {
public static void main(String[] args) {
int[][] arr = new int[2][2]; // here we have created a 2-D array
Scanner scanner = new Scanner(System.in);
for (int row = 0; row < arr.length; row++) {
System.out.println(“welcome to row number “+row);// this text is here so you can understand what row are we in.
for (int column = 0; column < arr[row].length; column++) {
System.out.println(“Enter the “+ column+ ” element of this row: “);
arr[row][column] = scanner.nextInt();
}
}
for (int row = 0; row < arr.length; row++) {
System.out.println(“Printing row :”+row);// this text is here so you can understand what row are we in.
for (int column = 0; column < arr[row].length; column++) {
System.out.print(arr[row][column] + ” “);
}
System.out.println();
}
}
}
Well, in the above program, there might be some things that might seem alien to you, and some things might be already understood. Let’s discuss the above program to get a more clear picture of what is happening.
So, first of all, we are creating the Scanner object so as to be able to get the user inputs for the array. Here, in order to input, we need to have two loops. The reason behind this is that one for loop is helping us go through each row, and another is helping us go to every element in the row. This is the reason why we named the variables in the for loop as row and column.
So, in short, here we are implementing the nested for loops.
Here, when the outer for loop runs once, the inner for loop runs until the condition is false (till it goes through all the elements in that row), and then again the outer for loop runs once, and then again the inner for loop runs until the condition is false, and this keeps going on, and finally, when the condition of the outer loop is false, we are out of the complete loop.
As you have observed, we are taking input inside the inner for loop. This is because, for every row, we have to fill all the available columns. For the first iterations, when the row = 0, which means that we are in the first row of the array, we are going to run the inner for loop twice, since there are two columns.
So, assuming such values of the column as 0 and 1(since at 2, the condition would be false),
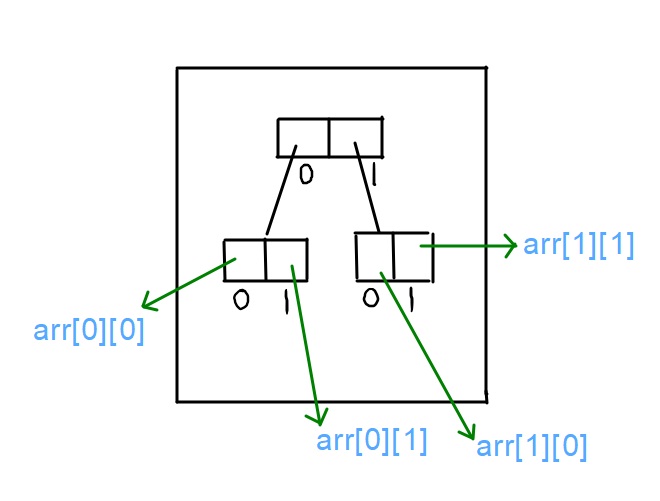
So, from the above diagram, it is quite easy to understand where are we going. See, doing arr[0] will take you to the first element in the array, which is itself an array. So, again we have to access its elements with help of its index. The same is the case with the other elements. You can also predict how many elements are there in the array, just by multiplying the number of rows by the number of columns. In the above example, we have 2*2 = 4 elements in the array. For example, If we have row = 2, and column=3, then the number of elements in the array is 2*3 = 6.
Earlier, there was mentioned that giving the number of rows is compulsory, but it is not the case with columns, which are optional. So, now let’s dive into why this is so.
Well, by this, I mean that it is fine if we write the instruction something like this –
int[][] x = [3][];
In the above instruction, we mean that the number of rows is going to be 3, but we are not specifying the size of the columns.
This is because we have seen that every element of the array is again an array, and an array may have a different element, so one row can have 2 columns, while the other row may have 5 columns, and the last row may have 3 columns. In short, the arrays can have a varying number of elements.
Have a look at the below program, where we have created such an array, with a different number of columns in each row. If you try executing it, the program is not going to have any errors, but we are not printing anything here.
public class ArrayWithDifferentColumns {
public static void main(String[] args) {
int[][] x={
{1, 2, 3, 4, 5},
{5, 6, 7},
{1, 2, 3, 4}
};
}
}
As you can see, in the above program, we had 3 elements in the array, and each element is again an array. Another amazing thing is that all the arrays have different numbers of elements, so this is like for every row, we are having a different number of columns if observed properly. For row number zero, there are 5 columns, row number one got 3 columns and row number two got 4 columns, which is why it is not compulsory to mention the number of columns while we create the array. But the thing is that it is compulsory to mention the number of rows because we are telling there how many elements you want to have in the array. If we say 3, there are going to be 3 elements in the array, and all the 3 elements are again arrays.
If the above explanation feels confusing, please try visualizing it. How is it going to be right if we did not specify how many elements are going to be there in the array? This is like you are going to a shop and asking for chocolate, but no telling how many chocolates you want. So how the shopkeeper is going to know this? This was a very informal example, but still… it quite relates.
Introduction to 3-dimensional arrays – 3D Array JAVA
Now, If you try 3-dimensional arrays, then it is like there is an array, which contains arrays, and those arrays also contain an array.
This just adds one more layer to the previous diagram. In the previous diagram, the arr[0][0] contains an element in the case of a 2D array, but in the case of a 3D array, the arr[0][0] will contain again an array, and so we will need to use its indexes to access the elements of that array. Well, we won’t go much into 3D arrays, but let’s understand how they are with help of an example. Let’s see the syntax, of how can we create a 3D array.
type[][][] arrayname = new int[size1][size2][size3];
So, we can conclude that the number of bracket pairs that we have after the type is the dimensions. Also, the syntax is similar to creating other arrays, but every time, we need to make changes according to the dimensions of our array.
Let’s directly visualize the 3D arrays, with an example array given below.
int [][][] x = new int[2][3][2];
The array created in the above example is going to have 12 elements, which is 2*3*2. This is quite straightforward.
So, if we do x[0], we get the first element of the array and find that at this index, there is present another array.
Again if we do x[0][0], we get the first element of the inner array, where there is again an array. So, again we need to use its index to access the array element.
So, then we do x[0][0][0]. This time, we got the element, which is inside an array, which is again inside an array. If it is confusing to read, let’s visualize it now.
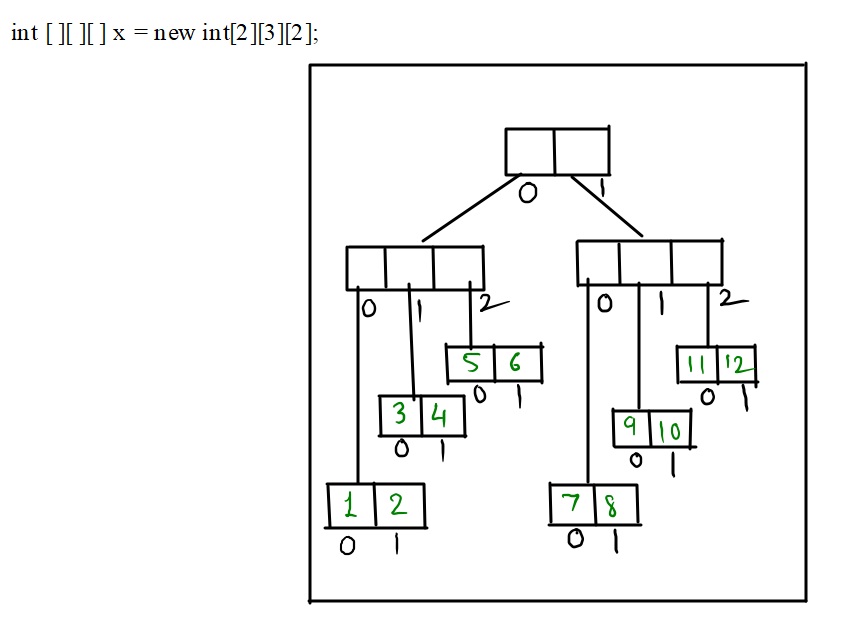
The above diagram is an illustration of how the layers of the 3D array are. The array contains arrays, which again contain arrays. Well, again as we increase the dimensions, the depth goes on increasing, like array inside the array and so on. So, it may become difficult to visualize.
So, this was about multidimensional arrays, and we discussed the 2-dimensional arrays. Hopefully, you have got the concept of multidimensional arrays or Array of arrays.
Some programs related to arrays –
- Write a program to search for an element in the array. If the element is found, print that the element was found, else print the element not found.
- Write a program for sorting the array(without using the sort method).
- Write a program for reversing the array.