In this article, we are going to understand and write a simple Java program, to find the largest and smallest numbers in the array. We would first understand how are we going to achieve this task, and then we are going to perform a Java program for the same. As a prerequisite for doing this program, you are required to have some knowledge about these concepts –
- Array in Java
- for loop in Java
- if else statements.
Largest and Smallest Number in Array Java
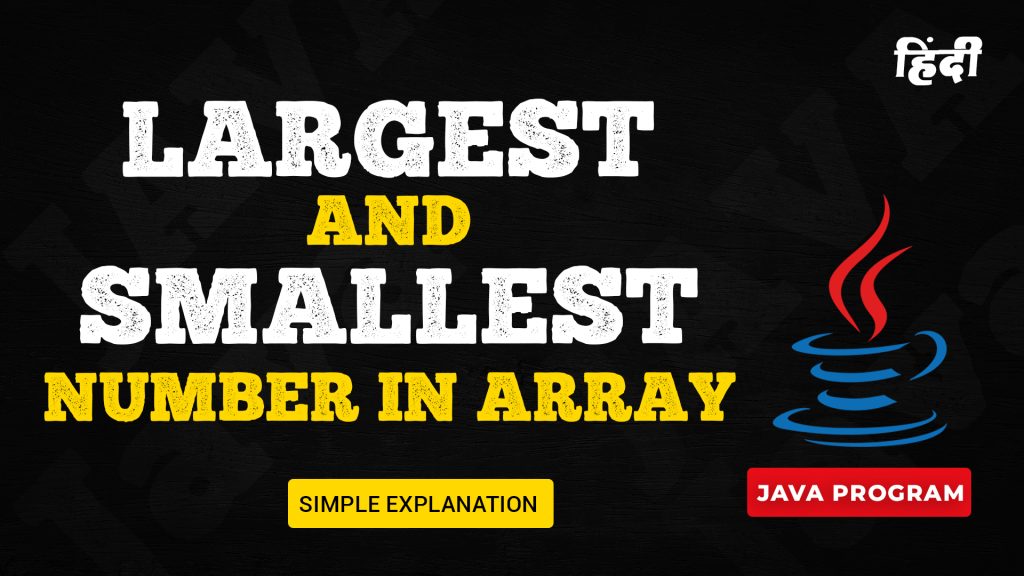
Program Approach –
First of all, let’s understand what are we going to do. We just need to find the smallest number and the largest number in the given array. We are going to see several approaches, through which, we can achieve our goals.
Let’s discuss them one by one –
Way 1 –
Sort the array in ascending order, and then the smallest element in the array is the first element in the array, and the largest element in the array is the last element in the array.
To sort the array, we are going to use the sort method, from the Arrays class. Let’s have a look at the program, which tries to demonstrate the same thing.
Let’s have a look at a simple example, through which, we can get more clear about this approach.
- Let’s say that the array given is – {40, 15, 27, 32, 31, 60, 57}
After sorting in ascending order, the array becomes {15, 27, 31, 32, 40, 57, 60} - In the sorted array, the smallest element in the array is the first element in the array, which is 15.
- In the sorted array, the largest element in the array is the last element in the array, which is 60.
Have a look at the below program, in which, we are trying to get the smallest and the largest element in the array, using the above approach.
import java.util.Arrays;
public class Example {
public static void main(String[] args) {
int[] numbers = new int[] {4, 15, 27, 32, 31, 66};
Arrays.sort(numbers);
// The smallest element in the array is going to be the first element in the sorted array.
System.out.println(“The smallest element in the array:” + numbers[0]);
// The largest element in the array is going to be the last element in the sorted array
System.out.println(“The largest element in the array:” + numbers[numbers.length – 1]);
}
}
As you can see in the above program, we have a simple array, and then we are using the sort method, from the Arrays class, to sort the array in ascending order. After that, we are printing the smallest and the largest element in the array. The smallest element in the sorted array is going to be the first element in the array, and the largest element in the sorted array is going to be the last element.
Let’s have a quick look at the output of the above program –
The largest element in the array:66
The smallest element in the array:4
This way, using the above simple approach, by sorting our array in ascending order, we can get the smallest and the largest number in the array.
Way 2 –
Manual approach –
In this approach, we are not going to sort the array. Instead, we are going to make some assumptions, and then we are going to compare the value with the other values in the array. In this array, we are going to assume that the largest element in the array is the first element in the array, and the smallest element in the array is also the first element in the array.
After that, we are going to loop through the array, and we are going to make some comparisons.
If the current element in the array is larger than the largest element in the array, then we would update our largest element to the new largest element. Similarly, if the current element is smaller than the assumed smallest element in the array, then we would update our smallest element to the new smallest element.
Have a look at the below program, through which, we can understand the above-discussed approach in a simple way –
public class Example {
public static void main(String[] args) {
int[] numbers = new int[] {4, 15, 27, 32, 31, 66};
// lets assume that the first element is the largest.
int largest = numbers[0];
// lets assume that the first element is the smallest.
int smallest = numbers[0];
for (int i = 0; i < numbers.length; i++) {
// update the smallest if we find smaller element than the assumed smallest element.
if (numbers[i] < smallest)
smallest = numbers[i];
// update the largest if we find larger element than the assumed largest element.
else if (numbers[i] > largest)
largest = numbers[i];
}
System.out.println(“The largest element in the array:” + largest);
System.out.println(“The smallest element in the array:” + smallest);
}
}
As you can see in the above program, we have a simple array, and then we are making an assumption, that the largest element in the array is the first element in the array, and the smallest element in the array is the first element in the array. After that, we are looping through the array and make some comparisons. If the current element is larger than the assumed largest, then we update our largest. We are doing something similar with the smallest element.
Let’s have a look at the output now –
The largest element in the array:66
The smallest element in the array:4
As you can see, we have the smallest, and the largest element in the array with us, using the manual approach. Here, we have made an assumption about the largest and the smallest elements in the array, and then we are looping through the array, making some comparisons.
Conclusion
In this article, we have understood how can we get the smallest and the largest element in the array in Java. We have also written some programs related to the approaches that we have discussed above. You can try those programs as well.
FAQ about Largest and Smallest Numbers in Array Java Program
Q: How to find the largest element in the array?
Ans: To get the largest element in the array, we just need to sort the array in ascending order, and the last element in the sorted array would be the largest element in the array. You can also sort the array in descending order, but in this case, the largest element would be the first element in the array.
Q: How to find the smallest element in the array in Java?
Ans: To get the smallest element in the array in Java, we can simply sort the array in ascending order, and the smallest element in the sorted array would be the first element in the array.
Q: How can we sort the integer array in Java?
Ans: To sort the integer array in java, we can make use of the sort method, from the Arrays class.