Celsius to Fahrenheit Conversion
In this article, we are going to understand and implement a simple Java program, to convert the temperature from degrees Celsius to Degrees Fahrenheit. This program is going to be a simple formula-based program, in which, we are going to get the temperature in degree Celsius from the user, and using the formula, we are going to convert it into degrees Fahrenheit.
So, the formula for converting temperature from degree Celsius to degree Fahrenheit is –
Celsius to Fahrenheit Conversion Formula
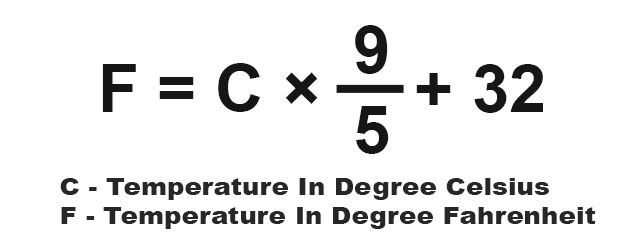
So, now let’s try to have a look at a simple Java program, through which, we would try to convert the temperature from degree Celsius to degrees Fahrenheit.
Steps for implementing the program –
Here is the set of steps that we are going to follow, in order to convert the temperature from degree Celsius to degrees Fahrenheit.
- Take the temperature in degrees Celsius as input from the user(or you can initialize yourself)
- Convert the temperature from degree Celsius to degree Fahrenheit using the above-mentioned formula.
- Show the temperature in degrees Fahrenheit as the output of our program.
So, as you can see that the steps are very easy to follow, so now, let’s have a look at the actual program now.
Java Program to Convert Celsius to Fahrenheit
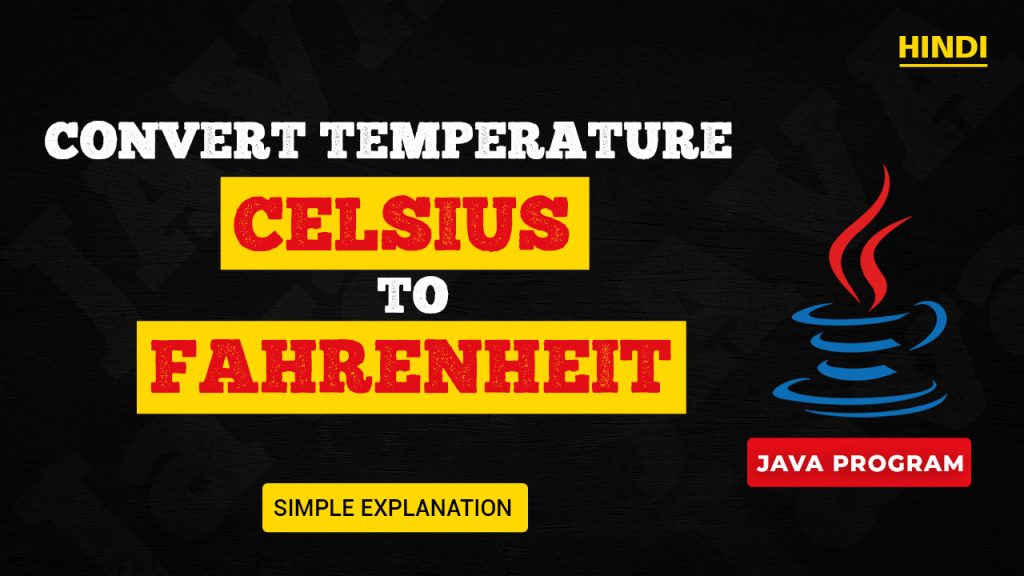
Now, let’s have a look at a simple Java program, to convert the temperature from degree Celsius to degrees Fahrenheit.
import java.util.Scanner;
public class Main
{
public static void main(String[] args) {
float celsius, fahrenheit;
// We are creating Scanner class object…
Scanner scanner = new Scanner(System.in);
System.out.print(“Please enter the temperature in degree Celsius:”);
// we are getting the user input value
celsius = scanner.nextFloat();
// converting the temperature from Celsius to Fahrenheit.
fahrenheit = (celsius * 9/5) + 32;
// displaying the output –
System.out.println(“the temperature in degree Fahrenheit is:” + fahrenheit);
}
}
As you can see in the above program, we are getting the temperature in degree Celsius from the user, and then we are just applying the simple formula to convert the temperature from degree Celsius to degrees Fahrenheit.
Let’s have a look at the output of the above program.
Output 1 –
Please enter the temperature in degrees Celsius:32
the temperature in degrees Fahrenheit is:89.6
Output 2 –
Please enter the temperature in degrees Celsius:39.7
the temperature in degrees Fahrenheit is:103.46001
As you can see from the output, we just need to provide the temperature in degrees Celsius, and we get the temperature in degrees Fahrenheit as the output.
Conclusion
We have understood and performed a simple Java program, to convert the temperature from degree celsius to degrees Fahrenheit. The program was very simple and formula based. In order to convert the temperature from degree celsius to degrees Fahrenheit, we just need to use the simple conversion formula stated above. You can also try to execute the above program, and also explore other programs.
FAQ about Java Program to Convert Celsius to Fahrenheit
Q: How to convert the temperature from degree Celsius to degrees Fahrenheit?
Ans: In order to convert temperature from degree Celsius to degrees Fahrenheit, we can use a simple conversion formula, f = (c * 9/5) + 32, where c is the temperature in degree celsius, and f is the temperature in degrees Fahrenheit.
Q: What is the formula for converting temperature from degree Celsius to degree Fahrenheit?
Ans: the formula for converting temperature from degree Celsius to degree Fahrenheit is f = (c * 9/5) + 32. Here, c is the temperature in degrees Celsius, and f is the temperature in degrees Fahrenheit.
Q: How much is 32 degree Celsius in degrees Fahrenheit?
Ans: 32 degrees Celsius is 89.6 degrees Fahrenheit.