Duck typing in Python
Duck typing is a very interesting concept, where the class or the type of the object is given lesser importance than the methods/attributes. Well, when we would consider some examples of the concept, you would simply get what it has to say. But this thing comes from the phrase – ‘If it quacks like a duck, and it walks like a duck, it must be a duck.
When we would consider examples for the same, you would get a clearer idea of what we are doing here.
Duck typing in Python
Let’s consider an example, which would get us to our way. In the below example, you can understand what we are actually doing. This example is just for giving you some understanding of what we mean by duck typing. After this, you would get an idea of what duck typing is. Have a look at the below example, and the explanation of the example is given after the example.
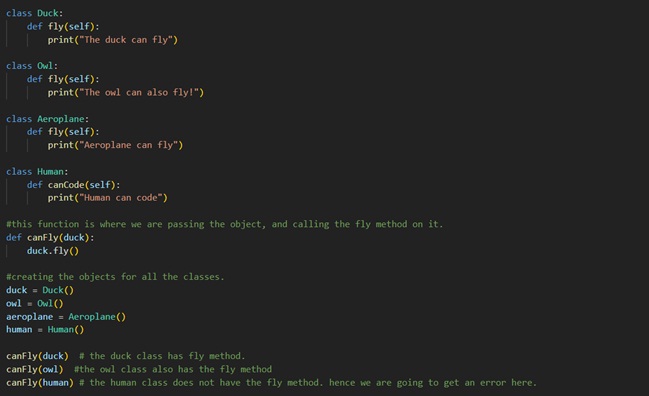
As you can see, here, we can find that there are some classes, like the Duck, Owl, Aeroplane, and Human. The first three classes have a method fly because they can fly. The Human class, however, is missing with the fly method. Also, we have a method, which is canFly, which would take an object basically. When we have written there a duck basically, we would pass a duck object to it first. But the thing is that we can pass any object here, and it that object belongs to such a class, which has the fly method, then we are not going to run into any problems.
But, when we are passing the human object to the canFly method, there is no fly method for the human, therefore it would give an error. You can try doing this program and the output is something like this in my case.
The duck can fly
The owl can also fly!
Traceback (most recent call last):
File “C:\Users\Administrator\Desktop\programs\duck.py”, line 30, in <module>
canFly(human) # the human class does not have the fly method. hence we are going to get an error here.
File “C:\Users\Administrator\Desktop\programs\duck.py”, line 20, in canFly
duck.fly()
AttributeError: ‘Human’ object has no attribute ‘fly’
As you can see, it says that the Human object has no attribute ‘fly’. So, what happening here is that we are passing in some object into the function canFly. Inside the function, we are calling the fly method on the object. So, it won’t get us into any problems, if that object has the fly method.
Now, the thing is that we need to have something more practical, so that we can understand where we are using the concept. So, now Let’s have a look at a more practical example here.
You might already be familiar with the len function in python. We use that method to get the length of several objects, like the list, string, tuple, set, and dictionary. So, we just call the len function and pass the required object there. Now, the thing is that the len function can be called on any object that defines the __len__() method.
So, when we are passing a valid object to the len function, it is returning the length of the object. Otherwise, we run into errors. Let’s have a look at the below example.
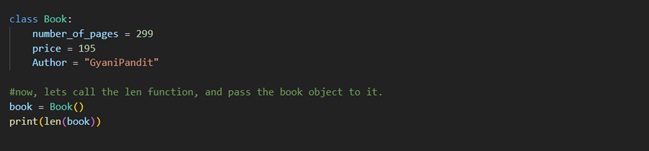
As you can see, we have a class Book, where we also got the number of pages, the price, and the author of the book. After that, we are creating the Book object, and then passing that book object to the len method. We have seen above, that we can call len function for any object, which defines the __len__() method. But here, the Book doesn’t have that method, so we are going to get into error this time.
We are going to get such an error message, saying that the Book object has no len().
But, when we define the __len__() method in the class Book, we can simply see that we can call it for the book as well. Have a look at the below example. Here, we are considering that the length of the book is the number of pages of the book.
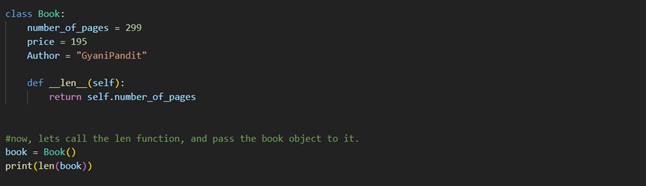
As you can see, we have defined the __len__() method for the Book this time, and we arse returning the number of pages of that particular book. So, we are simply going to get the number of pages of the book as a return value from the len function for the book.
Here, the bottom line lies in not binding the code to some specific datatype. The only constraint here to use the len function is that the __len__() method must be defined. The len method can be used with the string, list, dictionary, etc…
Thus, saying that If it quacks like a duck, and it walks like a duck, it must be a duck.