In this article, we are going to learn about a simple C program, where we are going to find the square root of some number. At times, it can be important for us to calculate the Square root of a given number, so it is important to learn how to do it.
In C language, it is very simple to calculate the square root of a given number. We just need to make use of the sqrt() function and provide the number to the function, to get the square root.
C Program to Find Square Root of a Number
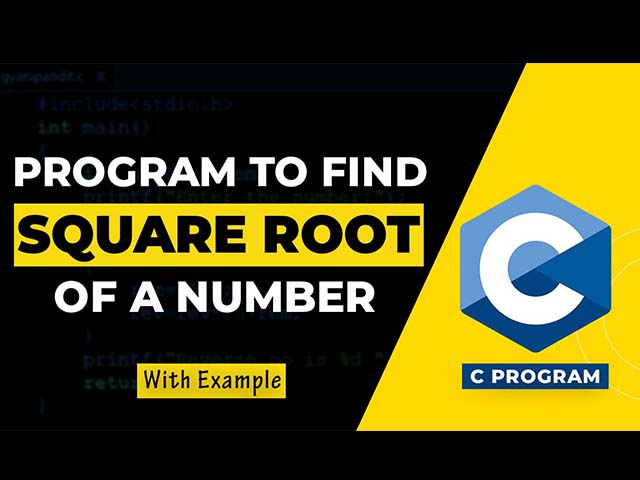
In this video, you can find the detailed explanation and program, to calculate the square root of the given number.
Here is the C program to calculate the square root of the given number –
#include <stdio.h>
#include <math.h>
int main() {
double number, squareRoot;
// Prompt the user to enter a number
printf(“Enter a number: “);
scanf(“%lf”, &number);
// Calculate the square root using the sqrt() function from the math library
if (number >= 0) {
squareRoot = sqrt(number);
printf(“The square root of %.2lf is %.2lfn”, number, squareRoot);
}
else {
printf(“Sorry, Square root of a negative number is undefined.n”);
}
return 0;
}
Output Case 1 (where the number is positive)
Enter a number: 144
The square root of 144.00 is 12.00
Output Case 2
Enter a number: 92
The square root of 92.00 is 9.59
Output Case 3 (Where the number is negative)
Enter a number: -10
Sorry, the Square root of a negative number is undefined.