C program to calculate Area of Rectangle
In this program, we are going to see how to calculate the area of rectangle program in c. So let’s start!
C Program to Calculate Area of Rectangle
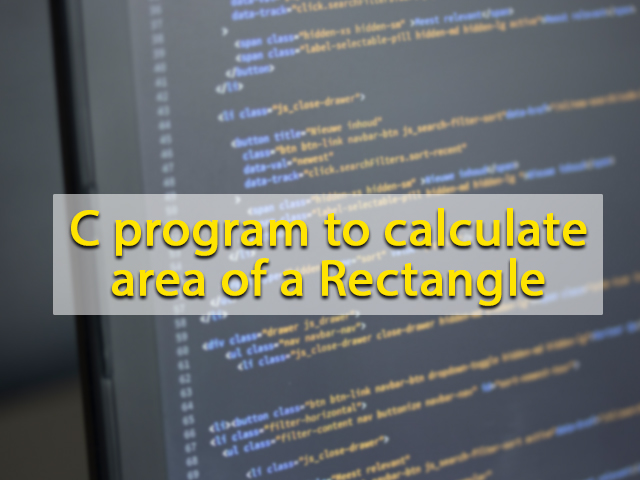
To calculate the area of the rectangle we need a formula first, that is :
Area = length * breadth
now we apply this formula to our program and we simply calculate the area of the rectangle let’s understand this with the help of the given program.
Method 1: using the standard formula.
//C program to find the maximum from the two numbers by using a user-defined function.
#include<stdio.h>
int main(){
int length, breadth, area;
printf(“Enter the length:”);
scanf(“%d”,&length);
printf(“Enter the breadth:”);
scanf(“%d”,&breadth);
area = length * breadth;
printf(“Area of rectangle is %d”, area);
return 0;
}
Output
Enter the length: 2
Enter the breadth: 4
The area of the rectangle is 8
Explanation:
- In the above program, we can see that there are three int-type variables to store the value of the length, breadth, and area of a rectangle.
- After that, we use Scanf to take user input in the form of length and breadth.
- Then we apply our formula and after that print the area of a rectangle that is set.
Method 2: By using a user-defined function.
// C program to calculate the area of a rectangle by using a user-defined function.
#include<stdio.h>
int area_rectangle(int length,int breadth){
return length * breadth;
}
int main(){
int length, breadth, area;
printf(“Enter length:”);
scanf(“%d”,&length);
printf(“Enter breadth:”);
scanf(“%d”,&breadth);
printf(“Area of rectangle is %d”,area_rectangle(length,breadth));
return 0;
}
Output
Enter length: 2
Enter breadth: 4
The area of the rectangle is 8
Step 1: To calculate the area of the rectangle we simply use the user-defined function from the above example, we can see that we create a user-defined function that gives the name area_rectangle and create two variables that is length and breadth, and it returns our area of a rectangle.
Step 2: Now in the next step we simply create a main function and create two variables to get length and breadth from user input.
Step 3: After that, we simply use printf() statement to get the area of a rectangle.
So in this way, we simply calculate the area of a rectangle. I hope you guys understand these programs.
So for now Keep exploring and keep learning!