Python Assignment Operators
Now, we are going to learn about the assignment operators in Python. Basically, these operators are used for assignment purposes. We are well familiar with one of the assignment operators, which is the simple assignment operator (=). We are going to use the assignment operators to assign values to the variables.
Here is the list of some assignment operators, many of which, we are going to explore soon.
Operator | Description | Example |
= | Simply assign the value | x = 3 (x is assigned value 3) |
+= | Add and Assign | x+=3 (add 3 to value of x and then assign the new value to x)
so, this is same as x = x+3 if x = 3, then new value of x is 6 with this operation |
– = | Subtract and Assign | x-=3 (subtract 3 from value of x and assign the new value to x)
so, this is same as x = x-3 if x =3, then new value of x is 0 |
*= | Multiply and Assign | x*=3 (multiply 3 to value of x and assign the new value to x)
this is same as x = x*3 if x = 3, then new value of x is 9 |
/= | Divide and Assign | x/=5 (Divide x by 5 and assign the new value to x)
this is same as x = x / 5. if x = 3 then new value of x is 0.6 |
//= | Divide and assign (floor value) | x//=5 (divide x by 5 and assign the floor value to the x)
this is same as x = x // 5. if x = 3 then new value of x is 0 |
**= | Exponentiate and assign | x**=2 (compute x to the power 2 and assign it to x)
this is same as x = x**2 if x =3 then new value of x is 9 |
%= | Perform division and assign the remainder of division to the variable. | x%=2 (divide x by 2 and assign remainder to x)
this is same as x = x%2 if x = 3 then new value of x is 1(this is the remainder when 3 is divided by 2) |
&= | Perform AND operation and assign to the variable | x&=2(perform AND operation between value of x and 2, and assign the result to x)
this is same as x = x&2 if x = 3 then new value of x is 2. (To understand this, you have to convert 3 and 2 in binary format and perform AND operation on them) |
|= | Perform OR operation and assign to the variable. | x|=2 (Perform OR operation between value of x and 2 and assign the result to x)
this is same as x = x|2 if x =3 then new value of x is 3. (To understand this, you need to convert 3 and 2 into binary format and perform OR operation on them) |
^= | Perform XOR operation and assign to the variable. | x^=2 (Perform XOR operation between value of x and 2 and assign the result to x)
this is same as x = x^2 if x = 3 then new value of x is 1 (To understand this, you need to convert 3 and 2 into binary format and perform XOR operation on them) |
>>= | Right shift and assign | x >> = 3 (perform right shift on x by 3 bit’s and assign the new value to x)
the right shift is again the operation on bit’s (binary digit’s). If x = 8 (1000), then the operation x >>=1 gives result 4(100) |
<<= | Left shift and assign | x<<=2 (perform left shift on x by 2 bit’s and assign the new value to x)
the left shift is again the operation on bit’s (binary digit’s). If x = 8 (1000), then the operation x <<=1 gives result – 16(10000) |
So, as you can see here, we have simply given some of the assignment operators in the above table, with symbol, and some examples. Now, let’s consider a simple example, which demonstrates the same things.
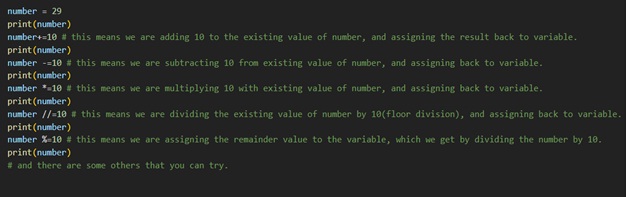
As you can see in the above program, we are simply assigning some value to the variable, which is number, and then we are using some of the different assignment operators, like +=, -=, *= and many others, and we are printing the respective output every time we are performing some operation.
So, now let’s try to have a look at the output –
29
39
29
290
29
9
As you can see, first we had assigned the value 29 to the variable number, and then we have used some different assignment operators, and we have got the respective outputs. We can use the assignment operators, as and when required. Now, let’s move on to the next type of operators.