Java Programming Course – Learn Java from Scratch
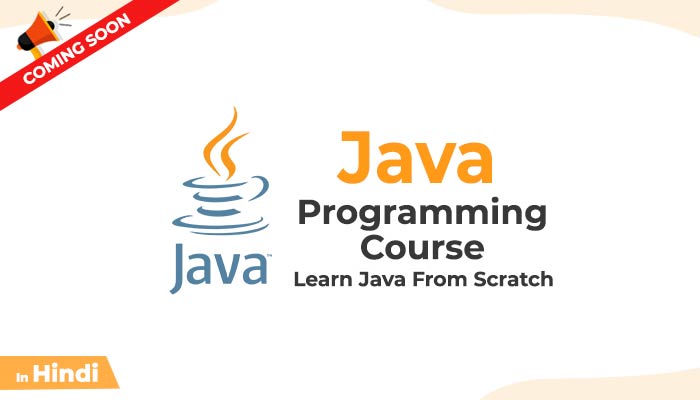
About Course
We welcome you to our course on Java programming language, which is one of the most popular and very widely used programming languages in the world. Learning Java can open many doors to learning and exploring different areas, like Android App Development, Desktop App development, Distributed Applications, Web-based applications, Game development, and much more.
We would consider many different concepts related to java from scratch and will go through different practical examples so that you can understand the concepts in an easy way. We do not assume that you are already familiar with some other programming language, but any prior knowledge related to programming would be appreciated.
We hope that you would enjoy learning Java with us. Happy learning!
What Will You Learn?
- Java fundamental concepts
- Practical examples
- Object oriented programming
- Multithreading
- And much more…
Course Content
Introduction
-
Introduction to Java
01:48 -
Java – terminologies and definitions
03:43 -
How does a Java program execute?
12:48 -
Download and install intellij IDEA and Java JDK
06:04 -
First Basic Program
07:17 -
Understanding the first Java Program
07:27
After Introduction
-
Data Types
15:37 -
Variables in java
12:16 -
Constants in java
00:00 -
Keywords
05:21 -
Understanding the bytecode
07:44 -
Understanding comments
07:06 -
typecasting
15:05 -
Taking user input
00:00
Operators in Java
-
Introduction to Operators
00:00 -
Arithmetic Operators
00:00 -
Assignment Operators
00:00 -
Comparison Operators
00:00 -
Logical Operators
00:00
Conditional Statements
-
Introduction to Conditional Statements
00:00 -
if else Statement
00:00 -
if else if Ladder
00:00 -
Nested if Statement
00:00 -
Using Logical Operators with if
00:00 -
Ternary Operator
00:00 -
Switch Case Statement
00:00
Looping in Java
-
Looping Introduction
00:00 -
Introduction to for Loop
00:00 -
What Happens When – for Loop
00:00 -
For Loop Program Example
00:00 -
Introduction to While Loop
00:00 -
What Happens When – While Loop
00:00 -
While Loop Program Example
00:00 -
Scope of Variables in While Loop
00:00 -
Introduction to do … While Loop
00:00 -
Do While Loop Program Example
00:00 -
Looping Program Example
00:00 -
Understanding Break Keyword
00:00 -
Understanding Continue Keyword
00:00
String in Java
-
Introduction to String
00:00 -
String Concatenation
00:00 -
Understanding Strings
00:00 -
Example Program for String
00:00
String in Java – String methods
-
Introduction to String Methods
00:00 -
toLowerCase Method
00:00 -
toUpperCase Method
00:00 -
charAt Method
00:00 -
Equals Method
00:00 -
Startswith Method
00:00 -
Endswith Method
00:00 -
Indexof Method
00:00 -
Replace Method
00:00 -
Trim Method
00:00 -
Strip Method
00:00 -
Split Method
00:00 -
Substring Method
00:00
Arrays in Java
-
Introduction to Array
00:00 -
How to Create an Array?
00:00 -
How to Access the Array Elements?
00:00 -
Arrays are Mutable
00:00
Methods from the Arrays Class
-
arrays method- toString
00:00 -
array method -sort
00:00 -
arrays method- fill
00:00
Arrays in Java – Introduction to Multidimensional Arrays
-
Creating 2 Dimensional Array
00:00
Math class Java
-
Math Class Introduction
00:00 -
abs method
00:00 -
cbrt method
00:00 -
ceil method
00:00 -
floor method
00:00 -
max method
00:00 -
min method
00:00 -
pow method
00:00 -
random method
00:00 -
sqrt method
00:00
Methods in Java
-
Methods Introduction
00:00 -
Methods Syntax
00:00 -
Example Program for Methods
00:00 -
method that takes no arguments and returns nothing
00:00 -
method that takes arguments but returns nothing
00:00 -
method that takes arguments and returns something
00:00 -
method that takes no argument but returns something
00:00 -
method overloading
00:00
Exception Handling in Java
-
introduction to exception handling
00:00 -
need of exception handling
00:00 -
exceptions vs error
00:00 -
exploring try and catch block(handling exception)
00:00 -
finally block
00:00 -
Exploring try-catch-finally
00:00 -
try with multiple catch blocks
00:00 -
throw keyword
00:00 -
throws keyword
00:00
StringBuilder in Java
-
introduction to stringbuilder
00:00 -
Using StringBuilder in an example program
00:00 -
length method
00:00 -
append method
00:00 -
deleteCharAt method
00:00 -
insert method
00:00 -
indexOf method
00:00 -
lastindexOf method
00:00 -
setCharAt method
00:00 -
reverse method
00:00 -
capacity method
00:00 -
trimtoSize method
00:00
File handling in Java
-
file handling introduction
00:00 -
creating a file object
00:00 -
creating a new file
00:00 -
getName method
00:00 -
length method
00:00 -
exists method
00:00 -
getabsolutepath and getpath method
00:00
File handling in Java – Writing to the file, using FileWriter
-
FileWriter Introduction
00:00 -
writing to a file – using FileWriter
00:00 -
appending data to the file – Using FileWriter
00:00 -
FileWriter – flush method
00:00 -
FileWriter – close method
00:00 -
what if you forget to close the resources
00:00
File handling in Java – Reading from the file, using FileReader
-
FileReader Introduction
00:00 -
reading data from a file
00:00 -
close method
00:00
File handling in Java – using scanner to read from the files
-
using scanner to read from the files
00:00
Object Oriented Programming
-
Introduction to Object Oriented Programming
00:00 -
Exploring classes and objects
00:00 -
Understanding the object creation
00:00 -
types of variables
00:00
Object Oriented Programming – Constructors
-
non parameterized constructor
00:00 -
parameterized constructor
00:00 -
constructor overloading
00:00
Object Oriented Programming – static keyword
-
static keyword – static variable
00:00 -
static keyword – static methods
00:00
Object Oriented Programming – access modifiers
-
access modifiers introduction
00:00 -
public access modifier
00:00 -
default access modifier
00:00 -
Protected access modifier
00:00 -
private access modifier
00:00
Object Oriented Programming – Principles of object oriented programming
-
introduction to OOP principles
00:00
Principles of object oriented programming – Inheritance
-
Inheritance introduction and some terminologies
00:00
Principles of object oriented programming – Types of inheritance
-
Types of inheritance – introduction to different types
00:00 -
single inheritance
00:00 -
multilevel inheritance
00:00 -
hierarchical inheritance
00:00
Principles of object oriented programming – Polymorphism
-
polymorphism introduction
00:00 -
Dynamic Polymorphism
00:00
Principles of object oriented programming – data abstraction
-
data abstraction
00:00
Principles of object oriented programming – encapsulation
-
encapsulation
00:00
Object Oriented Programming – this keyword
-
this keyword – using this keyword to refer the object variable
00:00 -
this keyword – using this keyword for constructor chaining
00:00 -
this keyword – using this to return the current object
00:00 -
this keyword – passing this as argument to method
00:00 -
this keyword – using this keyword to call some method
00:00 -
this keyword – passing this keyword as an argument to constructor
00:00
Object Oriented Programming – abstract classes part 1 & abstract class part 2
-
abstract classes part 1 & abstract class part 2
00:00
Object Oriented Programming – super keyword
-
super keyword – accessing the parent class variable
00:00 -
super keyword – using super to call the superclass method
00:00 -
super keyword – using super to call the parent class constructor
00:00
Object Oriented Programming – final keyword
-
final keyword – using final keyword with variables
00:00 -
final keyword – using final keyword with methods
00:00 -
final keyword- using final keyword with class
00:00
Object Oriented Programming – Understanding interface
-
interface introduction – what is an interface
00:00 -
how to create an interface
00:00 -
static and default methods in interface
00:00
Introduction to Multithreading in Java
-
What is multitasking
00:00 -
What is a thread
00:00 -
creating thread by extending Thread class
00:00 -
getId method
00:00 -
getName and setName method
00:00 -
getPriority and setPriority method
00:00 -
sleep method
00:00 -
getState method
00:00 -
creating thread using the runnable interface
00:00 -
Thread class or Runnable interface?
00:00