C++ Programming Course
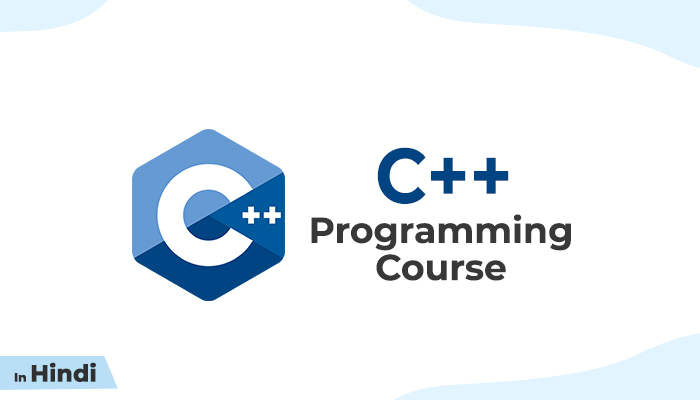
What Will You Learn?
- C++ Programming Course
Course Content
Introduction
-
Introduction
01:57
Installing VScode and compiler
-
Installing VScode and compiler
04:56
First program
-
First program
04:28
Datatypes
-
Datatypes
05:28
variables
-
variables
08:14
constants
-
constants
05:06
Keywords
-
Keywords
03:55
Identifiers
-
Identifiers
01:53
comments
-
comments
02:56
Operators
-
Operators in c++
02:39 -
Arithmetic operators
06:42 -
Assignment operators
04:05 -
Relational operators (Comparison)
06:53 -
Logical operators
06:12 -
Bitwise operators
16:29 -
Operator precedence and associativity
05:29
How to take user input in C++
-
How to take user input in C++
03:34
Control statements (if, else if, nesting of if else, switch), Ternary operator
-
Conditional statements in c++
02:04 -
if statements
08:52 -
if else ladder
07:31 -
nesting of if else
07:59 -
switch case statements
08:53 -
ternary operator
04:55
namespaces in C++
-
namespaces in C++
06:26
Looping statements
-
Introduction to Looping
00:55 -
for loop
05:05 -
while loop
04:55 -
do while loop
11:05 -
break keywords
04:08 -
continue keywords
04:06 -
nested loop
08:22
Arrays in C++
-
Introduction to Array in C++
01:34 -
What is an array?
00:00 -
how to take the user input in the array
06:30 -
How to access the element of an array ?
05:36 -
iterating through array
03:45 -
array program – linear search
08:09 -
array program – binary search
00:00 -
introduction to 2D arrays
00:00
String in C++
-
what are strings?
03:42 -
how to take the user input for strings ?
05:13 -
how to access the strings ?
03:04 -
strcat()
04:31 -
strcpy()
03:54 -
strcmp()
06:21 -
strlen()
04:11
Pointers
-
what are pointers?
02:46 -
how to create pointers?
04:35 -
how to traverse an array using pointers?
03:59 -
null pointer
04:12 -
void pointer
04:25 -
wild pointer
03:17
Functions
-
What is a function?
08:07
Lambda expression in C++
-
Lambda expression in C++
03:37
Structures
-
What is a structure
08:23
Union
-
What is union?
06:40
File handling
-
Introduction to file handling
04:09 -
How to read data from file
04:20 -
How to write data to a file
03:41 -
How to delete a file
02:14
Dynamic memory allocation
-
Dynamic memory allocation
11:32